Warning on Connecting to MongoDB with a Node server
Solution 1
Check your mongo version
mongo --version
If you are using version >= 3.1.0 change you mongo connection file to ->
MongoClient.connect("mongodb://localhost:27017/YourDB", {
useNewUrlParser: true,
useUnifiedTopology: true
})
For details about the useUnifiedTopology
option added in 3.2.1, see https://github.com/mongodb/node-mongodb-native/releases/tag/v3.2.1
Solution 2
My advice is to leave it as it is (maybe place a warning). The useUnifiedTopology: true
option does not work correctly.
More precisely, in the event of a loss of connection to the DBMS, it will never be restored. Current version 3.3.3 does not solve this problem.
Solution 3
I got the same error and resolved using the below template.
var MongoClient = require('mongodb').MongoClient
const client = new MongoClient(uri, {useUnifiedTopology: true});
client.connect().then((client)=>{
var db = client.db('db_name')
db.collection('collection_name').find().toArray(function (err, result) {
if (err) throw err
console.log(result);
})
})
This worked for me. and now it's not showing any DepricationWarning.
Solution 4
I want to add to this thread that it may also have to do with other dependencies.
For instance, nothing I updated or set for NodeJS, MongoDB or Mongoose were the issue - however - connect-mongodb-session
had been updated and starting slinging the same error. The solution, in this case, was to simply rollback the version of connect-mongodb-session
from version 2.3.0
to 2.2.0
.
UPDATE: The issue is now fixed in [email protected]
.
Solution 5
This is what made it work for me.
MongoClient.connect('mongodb://localhost:27017/blogdb', {useUnifiedTopology: true} , (err, client) =>
{
if (err) throw err;
const db = client.db('blogdb');
const collection = db.collection('posts');
const users = db.collection('users');
app.locals.collection = collection;
app.locals.users = users;
});
This is the same exact code I had before where I was getting the same deprecation message. The only difference is I haven't added the 'useUnifiedTopology: true' line.
MongoClient.connect('mongodb://localhost:27017/blogdb', (err, client) =>
{
if (err) throw err;
const db = client.db('blogdb');
const collection = db.collection('posts');
const users = db.collection('users');
app.locals.collection = collection;
app.locals.users = users;
});
Related videos on Youtube
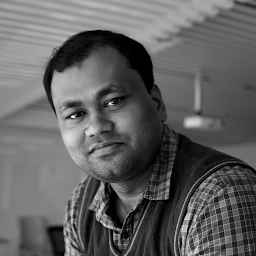
Momin
ES6, JavaScript with Vue/React? Yep, that's what I do best. Mix that with lots of head nodding from ambient music, and you've got me in a nutshell.
Updated on October 13, 2021Comments
-
Momin over 2 years
Connecting with MongoDB native driver
I wrote following code to connect mongodb through native driver which has been install with
npm install mongodb --save
const MongoClient = require("mongodb").MongoClient; const url = "mongodb://127.0.0.1:27017"; const dbName = "game-of-thrones"; let db; MongoClient.connect( url, { useNewUrlParser: true }, (err, client) => { if (err) return console.log(err); db = client.db(dbName); console.log(`Connected MongoDB: ${url}`); console.log(`Database: ${dbName}`); } );
When I write on the terminal
node server.js
I got following error(node:3500) DeprecationWarning: current Server Discovery and Monitoring engine is deprecated, and will be removed in a future version. To use the new Server Discover and Monitoring engine, pass option { useUnifiedTopology: true } to MongoClient.connect. Connected MongoDB: mongodb://127.0.0.1:27017 Database: game-of-thrones
The database is connected, but how can I get rid out from the warning
-
Greg over 4 yearsThis is fixed in version 2.3.1 Yay!
-
davidm_uk about 4 yearsThis issue is shown as fixed in driver version 3.3.5, so this should no-longer be a problem.
-
PrestonDocks almost 4 yearsUser wants to know about Native Mongo client
-
Native Coder over 3 years@davidm_uk can confirm, answer is no longer relevant. Won't down-vote because it was both relevant and correct at the time of its posting.
-
Native Coder over 3 yearsGreat call-out. Some people may not think to check their dependencies
-
Bae over 2 yearsThis does not really answer the question. If you have a different question, you can ask it by clicking Ask Question. To get notified when this question gets new answers, you can follow this question. Once you have enough reputation, you can also add a bounty to draw more attention to this question. - From Review