Webpack extreme slow build
Solution 1
One thing, specifically in development, make sure to set the mode
:
module.exports = {
mode: "development"
// Other options...
}
If not set at all (looks like it's not in the shared webpack config), it will default to "production"
. This is ideal since you'll want your code ran in production mode for deploying because it minifies, does dead code elimination, some packages (eg: React) ship different builds depending on the environment, among other things.
However, if you're in development, running in production mode can add some build time cost because doing this minification, dead code elimination, etc. add to the overall build time. This is unavoidable for the actual production built so this won't help that build time, but assuming you're building significantly more in development it should save some time.
Additionally, consider changing the devtool
option to a cheaper option such as cheap-module-eval-source-map
or one of the other options in the docs: https://webpack.js.org/configuration/devtool/. The docs explain the differences and build time costs. The current option source-map
is one of the slowest options.
module.exports = {
devtool: "eval-cheap-module-source-map"
// Other options...
}
Solution 2
Removing dev-tool: source-maps
should speed up the compilation time. Also it's important to note that your files are on the larger side as the output is over 1mb.
You could also add the cacheDirectory: true
flag to babel-loader. I found that this greatly sped up my builds at my company. Reference - https://webpack.js.org/loaders/babel-loader/#options
Also I personally use the --watch
flag for when I want webpack to run in watch mode. This lets me have more control over when I want it to actually run.
Solution 3
You could try:
devtool: 'eval',
It will produce a considerably larger file but in half the time. Not recomended for production.
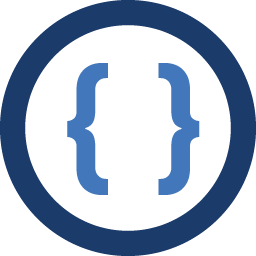
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I use webpack + typescript + react.
webpack.config.js is:
var webpack = require('webpack'); var path = require('path'); var node_modules_dir = path.join(__dirname, 'node_modules'); var deps = [ 'react/react.js', 'react-dom/react-dom.js', ]; var config = { devtool: 'source-map', context: __dirname + '/Scripts/ts', entry: { server: "./server.js", client: "./client.ts" }, output: { path: path.resolve(__dirname, "Scripts/public/"), filename: '[name].bundle.js' }, resolve: { alias: {}, modulesDirectories: ["node_modules"], extensions: ['', '.webpack.js', '.web.js', '.ts', '.tsx', '.js'] }, module: { noParse: [], // Use the expose loader to expose the minified React JS // distribution. For example react-router requires this loaders: [ { test: /\.ts(x?)$/, exclude: /(node_modules|bower_components)/, loader: 'babel-loader?presets[]=es2015&presets[]=react!ts-loader' }, { test: path.resolve(node_modules_dir, deps[0]), loader: "expose?React" }, ] }, watch: true }; deps.forEach(function (dep) { var depPath = path.resolve(node_modules_dir, dep); config.resolve.alias[dep.split(path.sep)[0]] = depPath; config.module.noParse.push(depPath); }); module.exports = config;
My problem is build speed. An initial process takes about 25s and incremental - 5-6 s. The result of:
webpack --profile --display-modules
is:
ts-loader: Using [email protected] and C:\Users\rylkov.i\Documents\Visual Studio 2013\Projects\react_test_app\react_test_app\tsconfig.json Hash: d6d85b30dfc16f19f4a6 Version: webpack 1.12.9 Time: 25547ms Asset Size Chunks Chunk Names client.bundle.js 1.14 MB 0 [emitted] client server.bundle.js 1.14 MB 1 [emitted] server client.bundle.js.map 1.31 MB 0 [emitted] client server.bundle.js.map 1.31 MB 1 [emitted] server [0] ./client.ts 80 bytes {0} [built] factory:38ms building:21905ms dependencies:1ms = 21944ms [0] ./server.js 70 bytes {1} [built] factory:14ms building:19ms = 33ms [1] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/~/expose-loader?Components!./components/index.js 179 bytes {0} {1} [built] [0] 33ms -> factory:2078ms building:8ms = 2119ms [2] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/Scripts/ts/components/index.js 210 bytes {0} {1} [built] [0] 33ms -> [1] 2086ms -> factory:19832ms building:4ms = 21955ms [3] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/Scripts/ts/components/main.tsx 4.78 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> factory:26ms building:331ms dependencies:1ms = 22313ms [4] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/~/react/react.js 172 bytes {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> [3] 357ms -> factory:315ms building:0ms = 22627ms [5] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/~/react/react.js 641 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> [3] 357ms -> [4] 315ms -> factory:1ms building:86ms = 22714ms [6] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/Scripts/ts/components/todoItem.tsx 2.81 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> factory:28ms building:576ms dependencies:70ms = 22629ms [7] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/Scripts/ts/components/selectControl.tsx 3.44 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> factory:27ms building:428ms dependencies:218ms = 22628ms [8] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/~/react-dom/react-dom.js 1.17 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> [7] 455ms -> factory:215ms building:4ms = 22629ms [9] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/Scripts/ts/components/selectItem.tsx 2.63 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> factory:28ms building:502ms dependencies:144ms = 22629ms [10] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/~/imports-loader?$=jquery!C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/Scripts/ui-select.js 99.6 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> [7] 455ms -> factory:150ms building:145ms = 22705ms [11] C:/Users/rylkov.i/Documents/Visual Studio 2013/Projects/react_test_app/react_test_app/~/jquery/dist/jquery.js 348 kB {0} {1} [built] [0] 33ms -> [1] 2086ms -> [2] 19836ms -> [7] 455ms -> [10] 295ms -> factory:10ms building:211ms = 22926ms
I think that this is extremely slow. react.js and react-dom.js are already compiled js files without extra require. My components are simply examples. One more problem with webpack is:
watch:true
attribute of config. I can't understand why is works not always. But maybe this is because slow build process. Thanks!