WebRTC videochat through Websockets
Solution 1
Have you looked at or come across WebRTC.io? It is an opensource GitHub project that utilizes Node.js and websockets to do the exact thing you are talking about. I, not being much of a javascript person, was able to figure out what it was doing within a week. It isn't a step by step instruction, but anyone with javascript experience would be able to figure out the function call order.
There are two bits to the code: the server side and the client side. The server side is run with Node.js, and serves up the client side code to the browser. If I remember correctly, since the two projects are separate, if you want to combine them you will have to copy the webrtcio.js file from the client side and paste it into the server side folder. Although, I think if you clone the github repository right, you might not have to worry about that.
Solution 2
You might want to have a look at the codelab I did for Google I/O: bitbucket.org/webrtc/codelab.
Step 5 shows how to set up a signaling server using socket.io, and Step 6 puts this together with RTCPeerConnection to make a simple video chat app.
You might also want to have a look at easyRTC (full stack) and Signalmaster (signaling server created for SimpleWebRTC).
The 'canonical' WebRTC video chat example at apprtc.appspot.com uses XHR and the Google App Engine Channel API for signaling.
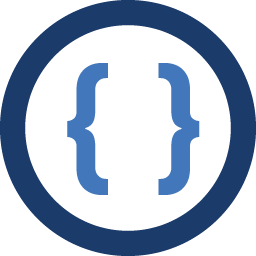
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm trying to develop a video-chat application using webRTC and WebSockets for signaling. My problem is that I don't know exactly what is the process of creating RTCPeerConnection and connect both peers(2 browsers) through webSocket(Locally at least).
I know how to communicate clients though WebSockets, but not with the RTCPeerConnection API, you know any tutorial step-by-step explaining the process?(Offer SDP, answers, ICE, ...) and, on the other hand, how looks the server code to managing these clients through RTCPeerConnection?
Here is my Node.js code for the server
"use strict"; // Optional. You will see this name in eg. 'ps' or 'top' command process.title = 'node-webrtc'; // Port where we'll run the websocket server var webSocketsServerPort = 1337; // websocket and http servers var webSocketServer = require('websocket').server; var http = require('http'); /* --------------------------------- GLOBAL VARIABLES ----------------------------------*/ // latest 100 messages //var history = [ ]; // list of currently connected clients (users) var clients = [ ]; /* --------------------------------- HTTP SERVER ----------------------------------*/ var server = http.createServer(function(request, response) { // Not important for us. We're writing WebSocket server, not HTTP server }); server.listen(webSocketsServerPort, function() { console.log((new Date()) + " Server is listening on port " + webSocketsServerPort); }); /* --------------------------------- WEBSOCKET SERVER ----------------------------------*/ var wsServer = new webSocketServer({ // WebSocket server is tied to a HTTP server. WebSocket request is just // an enhanced HTTP request. For more info http://tools.ietf.org/html/rfc6455#page-6 httpServer: server }); // This callback function is called every time someone // tries to connect to the WebSocket server wsServer.on('request', function(request) { console.log((new Date()) + ' Connection from origin ' + request.origin + '.'); // accept connection - you should check 'request.origin' to make sure that // client is connecting from your website // (http://en.wikipedia.org/wiki/Same_origin_policy) var connection = request.accept(null, request.origin); // we need to know client index to remove them on 'close' event var index = clients.push(connection) - 1; console.log((new Date()) + ' Connection accepted.'); // user sent some message connection.on('message', function(message) { for (var i=0; i < clients.length; i++) { clients[i].sendUTF(message); } }); // user disconnected connection.on('close', function(conn) { console.log((new Date()) + " Peer " + conn.remoteAddress + " disconnected."); // remove user from the list of connected clients clients.splice(index, 1); }); });