What's onCreate(Bundle savedInstanceState)
Solution 1
If you save the state of the application in a bundle (typically non-persistent, dynamic data in onSaveInstanceState
), it can be passed back to onCreate
if the activity needs to be recreated (e.g., orientation change) so that you don't lose this prior information. If no data was supplied, savedInstanceState
is null.
... you should use the onPause() method to write any persistent data (such as user edits) to storage. In addition, the method onSaveInstanceState(Bundle) is called before placing the activity in such a background state, allowing you to save away any dynamic instance state in your activity into the given Bundle, to be later received in onCreate(Bundle) if the activity needs to be re-created. See the Process Lifecycle section for more information on how the lifecycle of a process is tied to the activities it is hosting. Note that it is important to save persistent data in onPause() instead of onSaveInstanceState(Bundle) because the latter is not part of the lifecycle callbacks, so will not be called in every situation as described in its documentation.
Solution 2
onCreate(Bundle savedInstanceState)
you will get the Bundle
null when activity get starts first time and it will get in use when activity orientation get changed .......
http://www.gitshah.com/2011/03/how-to-handle-screen-orientation_28.html
Android provides another elegant way of achieving this. To achieve this, we have to override a method called onSaveInstanceState()
. Android platform allows the users to save any instance state. Instance state can be saved in the Bundle. Bundle is passed as argument to the onSaveInstanceState method.
we can load the saved instance state from the Bundle passed as argument to the onCreate
method. We can also load the saved instance state in onRestoreInstanceState
method. But I will leave that for the readers to figure out.
Solution 3
As Dhruv Gairola answered, you can save the state of the application by using Bundle savedInstanceState. I am trying to give a very simple example that new learners like me can understand easily.
Suppose, you have a simple fragment with a TextView and a Button. Each time you clicked the button the text changes. Now, change the orientation of you device/emulator and notice that you lost the data (means the changed data after clicking you got) and fragment starts as the first time again. By using Bundle savedInstanceState we can get rid of this. If you take a look into the life cyle of the fragment.Fragment Lifecylce you will get that a method "onSaveInstanceState" is called when the fragment is about to destroyed.
So, we can save the state means the changed text value into that bundle like this
int counter = 0;
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putInt("value",counter);
}
After you make the orientation the "onCreate" method will be called right? so we can just do this
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if(savedInstanceState == null){
//it is the first time the fragment is being called
counter = 0;
}else{
//not the first time so we will check SavedInstanceState bundle
counter = savedInstanceState.getInt("value",0); //here zero is the default value
}
}
Now, you won't lose your value after the orientation. The modified value always will be displayed.
Solution 4
onCreate(Bundle savedInstanceState) Function in Android:
When an Activity first call or launched then onCreate(Bundle savedInstanceState) method is responsible to create the activity.
When ever orientation(i.e. from horizontal to vertical or vertical to horizontal) of activity gets changed or when an Activity gets forcefully terminated by any Operating System then savedInstanceState i.e. object of Bundle Class will save the state of an Activity.
After Orientation changed then onCreate(Bundle savedInstanceState) will call and recreate the activity and load all data from savedInstanceState.
Basically Bundle class is used to stored the data of activity whenever above condition occur in app.
onCreate() is not required for apps. But the reason it is used in app is because that method is the best place to put initialization code.
You could also put your initialization code in onStart() or onResume() and when you app will load first, it will work same as in onCreate().
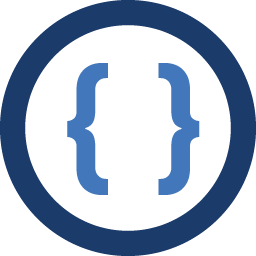
Admin
Updated on December 14, 2020Comments
-
Admin over 3 years
Can anyone help me to know about the
Bundle savedInstanceState
inonCreate(Bundle savedInstanceState)
I am newbie in Android. I try to understand it from developer.android.com. But I am not able to understand. Can anyone simplify it? -
Alex Lockwood almost 12 years
savedInstanceState
will be non-null
ifonSavedInstanceState()
was previously called. orientation changes are only one example in which this might occur. -
batbrat about 10 years@AlexLockwood, I'm trying to think of all the situations where this might occur. Is there any documentation? One instance I can think of is if the user switches apps and comes back, but the activity has been destroyed for recovering resources. In this case android will re-create the activity with a bundle if onSaveInsanceState() was overriden, right?
-
Alex Lockwood about 10 years@batbrat Yes... the comment I made (almost two years ago) is not entirely correct. I guess the best way to put it is the
Bundle
argument toonCreate(Bundle)
will be non-null
if and only if theActivity
had it'sonSaveInstanceState
method previously called. This happens during orientation changes... and also happens when the activity is coming back after being killed by the OS, and when you trigger any other configuration change of interest on your device. -
batbrat about 10 yearsThanks for the clarification Alex. It's good to have some clarity on the subject. I'd forgotten about configuration changes other than orientation switches in particular.
-
Francisco Corrales Morales over 9 yearsdoes other activities share the same Bundle ?, if I start another activity, does it pass the Bundle from the first one ?
-
laalaguer about 7 yearsNotice that this also holds true on Activity. Can you explain more on the difference of (Bundle of Activity) and Bundle of Fragment?
-
Asad about 7 yearsYou can get a clear understanding from the life cycles of activity and fragment where a activity can display multiple fragments at a time.So, the basic difference is that you may want to save some values for all the fragments of an activity, then u will use the bundle of activity otherwise u should use bundle of fragment if it is for specific fragment.