What's the difference between getline and std::istream::operator>>()?
Solution 1
The difference is thatstd::getline
— as the name suggests — reads a line from the given input stream (which could be, well, std::cin
) and operator>>
reads a word1.
That is, std::getline
reads till a newline is found and operator>>
reads till a space (as defined by std::isspace
) and is found. Both remove their respective delimiter from the stream but don't put it in the output buffer.
1. Note that >>
can also read numbers — int
, short
, float
, char
, etc.
Solution 2
the >>
operator of std::istream
read space separated strings.
getline
reads up to a given separator ('\n' as default).
So getline
admits a string containing spaces, the >> operator doesn't
Solution 3
Simple Answer: getline: As the name suggests, gets everything till it encounters a "\n" newline character. Here, input is always considered as a string. So, if you write:
string someStr;
getline(cin, someStr); //here if you enter 55, it will be considered as someStr="55";
">>" (Bitwise right shift Operator): Is smart and dumb at the same time. It will get input in any Basic Data types that you define, but it will stop at the first non Data Type char encountered. E.g.
int someInt;
std::cin >> someInt; //here if you enter "55some", >> will only consider someInt=55;
So, if you write:
string someStr;
cin >> someStr;
here, if you enter, "I am Batman", cin understands that "I" is a string, but " " (space is not), so it stops at I and you will get someStr="I".
IMP Point: If you use >> and getline back to back you will run into problems. for >>, even the "\n" (newline char) is not a Data type. So, if you write,
int someStr, someStr2;
std::cin >> someStr; //here you enter "Batman"
getline(cin, someStr2); //here you enter "Bruce"
since, >> does not recognize "\n" char, it will only see "Batman" and exit the buffer, leaving the "\n" for someStr2. So, someStr2 will not get "Bruce", but ""(new line char).
Solution 4
There are actually two getline
functions: one in the many stream
classes and one free function. The stream getline
functions take as a parameter a char* array into which it reads the characters and the max number of characters. The free function takes as a parameter a std::string
so it resizes the string when it is needed. Both functions can take optionally a third parameter, which is a delimiter ('\n'
by default).
The input operator operator>>
is for reading formatted input. For example, if you run int i; std::cin >> i;
it will try to convert the input into an integer whereas the getline
functions will read it s characters. The input operator also by default removes all white spaces. It is also used for custom serialisation of classes into strings as shown here.
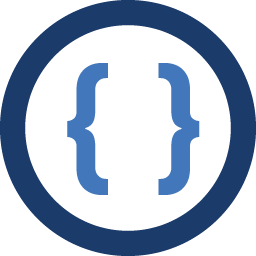
Admin
Updated on June 24, 2022Comments
-
Admin almost 2 years
#include <iostream> #include <string> using namespace std; int main() { string username; cout<< "username" ; cin >> username; }
So I was curious on what's the difference between these two codes, I heard it's the same thing but if it is then why two ways of doing it then?
#include <iostream> #include <string> using namespace std; int main() { string username; cout << "username" ; getline (cin,username) ; }
-
πάντα ῥεῖ almost 9 years"and operator>> reads a word." If applied to a
std::string
variable, yes. -
Svalbard about 3 yearsMore precisely, `operator >>' reads till a space or a newline character is found.
-
Nawaz about 3 years@Svalbard: That is not precise at all.
operator >>
reads till a space, as defined by std::isspace, is found. That is precise. Also,std::isspace
depends on the current locale, which could define anything to be a space. You can even define all the lowercase letters as space, thenoperator>>
would skip all lowercase letters.