What are Closures and Callbacks?
Closures have already been well handled in Stackoverflow here is just a selection:-
How does a javascript closure work?
What exactly does “closure” refer to in JavaScript?
can you say this is a right example of Javascript Closure.. Where the places we need to consider avoiding the closures??
JavaScript scope and closure
Javascript Closures and ‘this’ context
JavaScript - How do I learn about “closures” usage?
Callbacks are a simpler concept. A callback is basically where a function accepts another function as a parameter. At some point during execution the called function will execute the function passed as a parameter, this is a callback. Quite often the callback actually happens as an asynchronous event, in which case the called function may return without having executed the callback, that may happen later. Here is a common (browser based) example:-
function fn() { alert("Hello, World"); }
window.setTimeout(fn, 5000);
Here the function fn
is passed as a callback to the setTimeout
function. Set timeout returns immediately however 5 seconds later the function passed as a callback is executed.
Closures and callbacks
Quite often the reason that closures get created (either incidentally, accidentally or deliberately) is the need to create a callback. For example:-
function AlertThisLater(message, timeout)
{
function fn() { alert(message); }
window.setTimeout(fn, timeout);
}
AlertThisLater("Hello, World!", 5000);
(Please read some of the linked posts to grasp closures)
A closure is created containing in part the message
parameter, fn
is executed quite some time after the call to AlertThisLater
has returned, yet fn
still has access to the original content of message
.
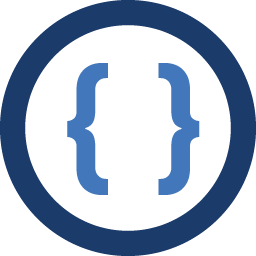
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
What are closures and callbacks in JavaScript? I've yet to find a good explanation of either.
-
Admin over 14 yearsAre callbacks most commonly used with setTimeout?
-
AnthonyWJones over 14 yearssetTimeout is a common usage but equally common would be
onreadystatechange
on theXmlHttpRequest
object. You will also see extensive usage of callbacks in code that uses Javascript frameworks such as JQuery. In many of these cases the callback is just where you would provide a little logic to customise a large but otherwise standard process. Also callbacks are frequently used to execute the same function over a set of objects.