What does '&&' operator indicate with { this.props.children && React.cloneElement(this.props.children, { foo:this.foo})
Solution 1
It's Short circuit evaluation
(if this part is true) && (this part will execute)
And it shorthand of:
if(condition){
(this part will execute)
}
Solution 2
The && is the exact same operator as you would find in any javascript expression, such as...
if( condition1 && condition2) {
}
It is a feature of javascript that an expression of the form...
(condition1 && condition2)
will evaluate to condition2, if condition1 is true, or null if condition1 is false. It is effectively shorthand for...
if(condition1) {
condition2;
}
We use this shorthand by placing a React element as condition 2, getting...
(condition1 && <ReactElement />)
which is effectively...
if(condition1) {
<ReactElement />
}
Solution 3
When && and || are used in this way, they are nicknamed "short circuit operators". In this usage, it can be thought of as a quickie "if (something is true)". So, if this.props.children is not null, it will call React.cloneElement. If it is null, it will not call React.cloneElement.
Here is a link on the official React documentation, with further reading: https://facebook.github.io/react/docs/conditional-rendering.html#inline-if-with-logical-ampamp-operator
Solution 4
In simple words the purpose of that &&
is:
Do not attempt to clone and render children when there are no children.
So if you use Users
like this:
<Users>
<Child1 />
<Child2 />
</Users>
then both Child1
and Child2
will get rendered with additional props foo
.
But if the parent is used in this way <Users/>
or <Users></Users>
, there are no child components to render. So we are performing a check before we invoke React.cloneElement
.
&&
is equivalent to boolean AND
: 1 AND A === A
=> 1 && A = A
||
is equivalent to boolean OR
: 1 OR A = 1
=> 1 || A = 1
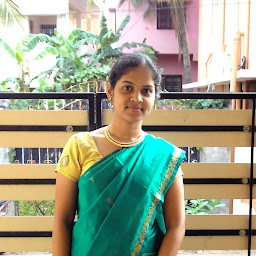
Arathi Arumugam
Updated on October 26, 2022Comments
-
Arathi Arumugam over 1 year
I have react class that is rendered using react router. I understand that React.cloneElement is used to pass elements from parent to child. But why/what does the '&&' operator do with this kind of statement :
class Users extends React.Component { getInitialState() { return { page:0 } }, foo(){ this.setState({'page':1}) } render() { return ( <div> <h2>Users</h2> { this.props.children && React.cloneElement(this.props.children, { foo:this.foo}) </div> ) } }
I would like to understand why are we using '&&' operator here.
-
TmSmth almost 5 yearsSo
condition2
is not really a condition as it depends only oncondition1
? -
sigmapi13 over 3 yearsLooks like it saves having to do :null for ternaries
-
squnk almost 2 yearsIs this only a react thing?