What does ${} (dollar sign and curly braces) mean in a string in JavaScript?
Solution 1
You're talking about template literals.
They allow for both multiline strings and string interpolation.
Multiline strings:
console.log(`foo
bar`);
// foo
// bar
String interpolation:
var foo = 'bar';
console.log(`Let's meet at the ${foo}`);
// Let's meet at the bar
Solution 2
As mentioned in a comment above, you can have expressions within the template strings/literals. Example:
const one = 1;
const two = 2;
const result = `One add two is ${one + two}`;
console.log(result); // output: One add two is 3
Solution 3
You can also perform Implicit Type Conversions with template literals. Example:
let fruits = ["mango","orange","pineapple","papaya"];
console.log(`My favourite fruits are ${fruits}`);
// My favourite fruits are mango,orange,pineapple,papaya
Related videos on Youtube
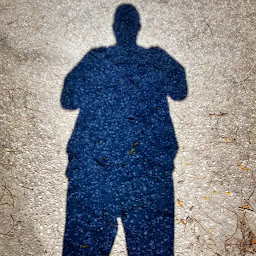
Darren Joy
BY DAY: Carbon based bag of water. BY NIGHT: Interested in learning, and sharing information about things learned. So much to do, so little time!
Updated on April 30, 2022Comments
-
Darren Joy about 2 years
I haven't seen anything here or on MDN. I'm sure I'm just missing something. There's got to be some documentation on this somewhere?
Functionally, it looks like it allows you to nest a variable inside a string without doing concatenation using the
+
operator. I'm looking for documentation on this feature.Example:
var string = 'this is a string'; console.log(`Insert a string here: ${string}`);
-
Admin about 8 years
-
Admin about 8 yearsThis isn't a bad question. It's a new feature, and I sure can't find a duplicate on SO, though an example of what exactly was seen would've been good.
-
RobG about 8 years@squint—agree, but sample code and reference to the source would have been helpful.
-
Darren Joy about 8 yearsExample added. Reference was in a coding challenge but it was a given, as if it was something you just use. Hadn't seen anything on it and couldn't find anything either. (Over the last several years I've never had to ask a Q here. SO just seems to have almost everything now...)
-
-
Sydney over 7 yearsOne thing that confused me for a long time is that template literals uses backtick, which is on the left of "1" on keyboard, instead of single quotation mark (').
-
T4NK3R over 6 yearsCool: You can even "inject" code:
console.log(`Your array:\n ${arr.join('\n ')}`)
-
Muhammad Musavi about 5 yearsIt's weird that
Template Literal
is not supported in IE or maybe it's natural for IE not to support cool things. Read more -
carloswm85 about 4 yearsIs it correct to say that template strings can be used to inject code into the string?
-
Joel H about 4 yearsYou're injecting expressions and expressions are code. You can't inject any type of code, however, only JavaScript expressions.
-
carloswm85 about 4 yearsI will try to be more specific. When using string literals I can inject expressions like obj.value INSIDE any string using the ${ } notation. Right?
-
Joel H about 4 yearsYes, and you can try it out for yourself in the console, e.g.
hey ${obj.name}
-
user3015682 almost 4 yearsSeems to be an alternative to string concatenation console.log("Let's meet at the "+foo);
-
pozzy over 3 years@Sydney just wanted to let you know that i was so confused why my string interpolation wasn't working until i saw your comment about the backtick. THANK YOUUU!!