What does while(*pointer) means in C?
Solution 1
while(x) {
do_something();
}
will run do_something()
repeatedly as long as x
is true. In C, "true" means "not zero".
'\0'
is a null character. Numerically, it's zero (the bits that represents '\0'
is the same as the number zero; just like a space is the number 0x20 = 32).
So you have while(*pointer != '\0')
. While the pointed-to -memory is not a zero byte. Earlier, I said "true" means "non-zero", so the comparison x != 0
(if x
is int
, short
, etc.) or x != '\0'
(if x
is char
) the same as just x
inside an if, while, etc.
Should you use this shorter form? In my opinion, no. It makes it less clear to someone reading the code what the intention is. If you write the comparison explicitly, it makes it a lot more obvious what the intention of the loop is, even if they technically mean the same thing to the compiler.
So if you write while(x)
, x
should be a boolean or a C int that represents a boolean (a true-or-false concept) already. If you write while(x != 0)
, then you care about x
being a nonzero integer and are doing something numerical with x
. If you write while(x != '\0')
, then x
is a char
and you want to keep going until you find a null character (you're probably processing a C string).
Solution 2
*pointer
means dereference the value stored at the location pointed by pointer
. When pointer
points to a string and used in while
loop like while(*pointer)
, it is equivalent to while(*pointer != '\0')
: loop util null terminator if found.
Solution 3
Let's start with a simple example::
int a = 2 ;
int *b = &a ;
/* Run the loop till *b i.e., 2 != 0
Now, you know that, the loop will run twice
and then the condition will become false
*/
while( *b != 0 )
{
*b-- ;
}
Similarly, your code is working with char*
, a string.
char var[10] ;
/* copy some string of max char count = 9,
and append the end of string with a '\0' char.*/
char *pointer = &var ;
while( *pointer != '\0' )
{
// do something
// Increment the pointer 1 or some other valid value
}
So, the while loop will run till *pointer
don't hit '\0'
.
while( *pointer )
/* The above statement means the same as while( *pointer != '\0' ),
because, null char ('\0') = decimal value, numeric zero, 0*/
But the usage can change when you do, while(*pointer != 'x'), where x can be any char
. In this case, your first code will exit after *pointer hits the 'x' char
but your second snippet will run till *pointer hits '\0' char
.
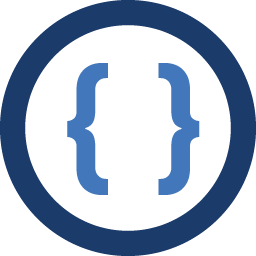
Admin
Updated on August 25, 2020Comments
-
Admin over 3 years
When I recently look at some passage about C pointers, I found something interesting. What it said is, a code like this:
char var[10]; char *pointer = &var; while(*pointer!='\0'){ //Something To loop }
Can be turned into this:
//While Loop Part: while(*pointer){ //Something to Loop }
So, my problem is, what does *pointer means?