What is an Object in Python?
Solution 1
To go deep, you need to understand the Python data model.
But if you want a glossy stackoverflow cheat sheet, let's start with a dictionary. (In order to avoid circular definitions, let's just agree that at a minimum, a dictionary is a mapping of keys to values. In this case, we can even say the keys are definitely strings.)
def some_method():
return 'hello world'
some_dictionary = {
"a_data_key": "a value",
"a_method_key": some_method,
}
An object, then, is such a mapping, with some additional syntactic sugar that allows you to access the "keys" using dot notation.
Now, there's a lot more to it than that. (In fact, if you want to understand this beyond python, I recommend The Art of the Metaobject Protocol.) You have to follow up with "but what is an instance?" and "how can you do things like iterate on entries in a dictionary like that?" and "what's a type system"? Some of this is addressed in Skam's fine answer.
We can talk about the python dunder methods, and how they are basically a protocol to implementing native behaviors like sized
(things with length), comparable types (x < y), iterable types, etc.
But since the question is basically PhD-level broad, I think I'll leave my answer terribly reductive and see if you want to constrain the question.
Solution 2
Everything is an object
An object is a fundamental building block of an object-oriented language. Integers, strings, floating point numbers, even arrays and dictionaries, are all objects. More specifically, any single integer or any single string is an object. The number 12 is an object, the string "hello, world" is an object, a list is an object that can hold other objects, and so on. You've been using objects all along and may not even realize it.
Objects have types
Every object has a type, and that type defines what you can do with the object. For example, the int
type defines what happens when you add something to an int, what happens when you try to convert it to a string, and so on.
Conceptually, if not literally, another word for type is class. When you define a class, you are in essence defining your own type. Just like 12
is an instance of an integer, and "hello world"
is an instance of a string, you can create your own custom type and then create instances of that type. Each instance is an object.
Classes are just custom types
Most programs that go beyond just printing a string on the display need to manage something more than just numbers and strings. For example, you might be writing a program that manipulates pictures, like photoshop. Or, perhaps you're creating a competitor to iTunes and need to manipulate songs and collections of songs. Or maybe you are writing a program to manage recipes.
A single picture, a single song, or a single recipe are each an object of a particular type. The only difference is, instead of your object being a type provided by the language (eg: integers, strings, etc), it is something you define yourself.
Solution 3
In the context of Python and all other Object Oriented Programming (OOP) languages, objects have two main characteristics: state and behavior.
You can think of a constructor as a factory that creates an instance of an object with state and behavior.
State - Any instance or class variables associated to that object.
Behavior - Any instance or class methods
The following is an example of a class, in Python, to illustrate some of these concepts.
class Dog:
SOUND = 'woof'
def __init__(self, name):
"""Creates a new instance of the Dog class.
This is the constructor in Python.
The underscores are pronounced dunder so this function is called
dunder init.
"""
# this is an instance variable.
# every time you instantiate an object (call the constructor)
# you must provide a name for the dog
self._name = name
def name(self):
"""Gets the name of the dog."""
return self._name
@classmethod
def bork(cls):
"""Makes the noise Dogs do.
Look past the @classmethod as this is a more advanced feature of Python.
Just know that this is how you would create a class method in Python.
This is a little hairy.
"""
print(cls.SOUND)
Though I agree with the comments that this question is a little vague. Please be a little more specific but the answer I've provided is a quick overview of classes and objects in Python.
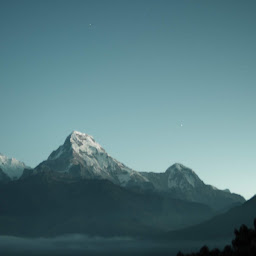
jchua
Updated on June 06, 2022Comments
-
jchua almost 2 years
I am surprised that my question was not asked (worded like the above) before. I am hoping that someone could break down this basic term "object" in the context of a OOP language like Python. Explained in a way in which a beginner like myself would be able to grasp.
When I typed my question on Google, the first post that appears is found here.
This is the definition: An object is created using the constructor of the class. This object will then be called the instance of the class.
Wikipedia defines it like this: An object is an instance of a Class. Objects are an abstraction. They hold both data, and ways to manipulate the data. The data is usually not visible outside the object.
I am hoping someone could help to break down this vital concept for me, or kindly point me to more resources. Thanks!
-
gmds almost 5 years
self.SOUND
would create an instance variable. -
kojiro almost 5 yearsHeh, I'm upvoting this for covering state and behavior and in particular instances of objects, which are the most elementary examples, and I'm slightly annoyed at myself for not covering that in my answer.
-
jchua almost 5 yearsThanks Skam, appreciate this answer too! Sorry was not able to define my question more appropriately.
-
jchua almost 5 yearsHi kojiro, this helps point in the direction whereby I can read up more on (especially on Python's data mode) - was already reading about this in Mark Lutz's book, however, was not able to draw the connections. However, your answer has helped confirm my direction. Thanks much.
-
jchua almost 5 yearsHi Bryan, this helps alot too, appreciate your answer!
-
Yaroslav Nikitenko about 4 yearsThank you. From the link I can see that a function, an object method, a class and a module are all objects. Do you know examples of what is not an object? (
type
seems to be also an object, maybe something has changed from Python 2?) -
Yaroslav Nikitenko about 4 yearsThank you. Are there examples of what is not an object in Python? (maybe in Python 2 there were some non-objects?)
-
kojiro about 4 yearsThere isn't really anything reference-able in Python that is not an object. I don't really think this has changed substantially since Python 2.
-
Rotkiv over 3 yearsstackoverflow.com/questions/865911/… Everything is an object
-
rdelmar about 3 years__init__ is not the constructor. You can't pass self into a constructor, since the constructor creates the object that self refers to. The instance is created by __new__, and __init__ initializes the attributes.