What is difference between "as" and "is" operator in Kotlin?
Solution 1
is X
is the equivalent of instanceof X
foo as X
is the equivalent of ((X) foo)
Additionally, Kotlin performs smart casting where possible, so no additional cast needed after you check the type using is
:
open class Person : A() {
val foo: Int = 42
}
open class A
and then:
if (p is Person) {
println(p.foo) // look, no cast needed to access `foo`
}
Solution 2
is
is type checking. But Kotlin has smart cast which means you can use a
like Person
after type check.
if(a is Person) {
// a is now treated as Person
}
as
is type casting. However, as
is not recommended because it does not guarantee run-time safety. (You may pass a wrong object which cannot be detected at compiled time.)
Kotlin has a safe cast as?
. If it cannot be casted, it will return null instead.
val p = a as? Person
p?.foo()
Solution 3
"Kotlin in action" by Dmitry Jemerov and Svetlana Isakova has a good example of as
and is
:
Solution 4
is - To check if an object is of a certain type
Example:
if (obj is String) {
print(obj.length)
}
as - To cast an object to a potential parent type
Example:
val x: String = y as String
val x: String? = y as String?
val x: String? = y as? String
Reference: https://kotlinlang.org/docs/reference/typecasts.html
Solution 5
as
is used for explicit type casting
val p = a as Person;
is
is exactly the same as instanceof
in Java. Which is used to check if an object is an instance of a class
if(a is Person) {
// a is an instance of Person
}
You can also used !is
as is it not an object of a class
fun cast(a: A) {
if(a is Person) {
val p = a as Person;
}
}
Related videos on Youtube
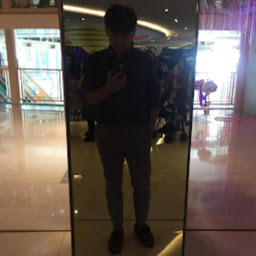
Shawn Plus
Updated on April 21, 2022Comments
-
Shawn Plus about 2 years
In Java, I can write code like:
void cast(A a) { if(a instanceof Person) { Person p = (Person) a; } }
In Kotlin, what should I do? Use
as
operator oris
operator? -
Shawn Plus over 6 yearsso if i always use 'is', that mean I can never use 'as' right? because IDE has smart cast
-
Joshua over 6 years@ShawnPlus It depends on your use case.
as?
is better if you use the variable in multiple place. Smart cast only work within the bracket only. -
Shawn Plus over 6 yearswe can use 'if' Statement to avoid ClassCastException, why need 'as'? , I can always use 'if' and 'is'
-
juzraai almost 6 yearsPlease edit your answer and add explanation to your code.
-
wonsuc almost 4 yearscan I see the any evidence reference of
as is not recommended
? -
Sourav Kannantha B almost 3 years@Joshua smart cast works outside bracket, if inside bracket returns. Eg:
if(a !is String) return //after this line, a is smart casted to string
-
Adeel Ahmad over 2 years@wonsuc
ClassA as ClassB
will throw exception if cast is not possible. HoweverClassA as? ClassB
will returnnull
if cast is not possible