what is dispatchEvent in Flash AS3?
Solution 1
An example might help? If you have Flash IDE, try this in your timeline:
var ball:Shape = new Shape();
ball.graphics.beginFill(0xFF0000);
ball.graphics.drawCircle(0, 0, 30);
ball.graphics.endFill();
addChild(ball);
stage.addEventListener(MouseEvent.MOUSE_MOVE, mouseMoveListener);
addEventListener("myCustomEvent", myCustomEventListener);
function mouseMoveListener(event:MouseEvent):void
{
dispatchEvent(new Event("myCustomEvent"));
}
function myCustomEventListener(event:Event):void
{
ball.x = stage.mouseX;
ball.y = stage.mouseY;
}
This is code demonstrates how the addEventListener and dispatchEvent are counterparts. The MOUSE_MOVE event is dispatched internally, but you can dispatch your own events just like the MOUSE_MOVE using dispatchEvent.
What happens in this code is that a MOUSE_MOVE from the stage is detected, but rather than handle that in the mouseMoveListener, you dispatch another event (called myCustomEvemt) which is handled in the myCustomEventListener instead. It works just like the MOUSE_MOVE event, only you dispatched the event instead of the flash player.
Hope this helps.
Solution 2
Say there are two classes : A and B . Now you want that "functionAfterMyFun" should occur after myFun has occured in class B. Then you would use dispatchEvent in class B's function myfun().
class A
{
function A()
{
objectOfB.addEventListener( "myFun_Happened", functionAfterMyFun)
}
function functionAfterMyFun()
{
//do something after myFun has happened in class B
}
}
class B
{
function myfun()
{
dispatchEvent(new Event("myFun_Happened"));
}
}
Solution 3
Basically it's a mechanism to notify objects that something has happened. When you dispatch an event, something needs to be listening for that event.
It's basically like making a telephone call ( dispatchEvent(new CallEvent(CallEvent.CALL)) ). If the person you are trying to ring isn't in, they aren't listening to the telephone event, so they can't do anything about it. If they are in ( addEventListener(CallEvent.Call, answerHandler) ), they can answer it, maybe send it straight to voice mail.
For a full rundown, try reading this article from adobe.
http://www.adobe.com/devnet/actionscript/articles/event_handling_as3_02.html
A lot of programming languages make use of events so it's very worth while getting to grips with it.
James
Response to Edit1
It looks to me like you have a method that has been set up to handle a MouseEvent.Click on some object somewhere. When that happens it sets another objects scaleX property to 0 (making it invisible). A new Event is then Dispatched with the type set to "setVolume". This on it's own wouldn't do anything. Somewhere else this is probably a line looks a little like addEventListener("setVolume", doSomethingMethod); . When the original method is fired (seemingly a mouse click), the doSomethingMethod should fire.
Related videos on Youtube
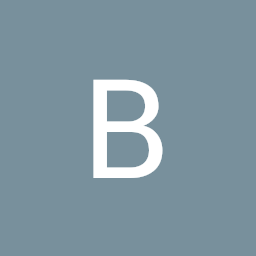
Comments
-
Bojan Muvrin almost 2 years
Hi all i want to know what is dispatchEvent in AS3. I didn't get any idea while Googling it. :( So please help me
Edit 1:
public static const SET_VOLUME:String = "setVolume"; private function onclick(evt:MouseEvent):void { soundClip.scaleX = 0; dispatchEvent(new Event(SET_VOLUME)); }
what does this means?? (
-
alecmce@coderex, Please mark your questions as answered when they are answered!
-
-
Papa De Beau over 10 years1067: Implicit coercion of a value of type String to an unrelated type flash.events:Event. :( Am I missing an import?
-
Vishwas over 10 yearsah sorry, there was a typo. I just edited it again. dispatchEvent ( new Event ("myFun_Happened"));
-
AturSams over 4 yearsGood explanation! Short and to the point. So basically anyone who is interested and has access to the Event Dispatcher object can listen to that object.