What is the best Java email address validation method?
Solution 1
Apache Commons is generally known as a solid project. Keep in mind, though, you'll still have to send a verification email to the address if you want to ensure it's a real email, and that the owner wants it used on your site.
EDIT: There was a bug where it was too restrictive on domain, causing it to not accept valid emails from new TLDs.
This bug was resolved on 03/Jan/15 02:48 in commons-validator version 1.4.1
Solution 2
Using the official java email package is the easiest:
public static boolean isValidEmailAddress(String email) {
boolean result = true;
try {
InternetAddress emailAddr = new InternetAddress(email);
emailAddr.validate();
} catch (AddressException ex) {
result = false;
}
return result;
}
Solution 3
Apache Commons validator can be used as mentioned in the other answers.
pom.xml:
<dependency>
<groupId>commons-validator</groupId>
<artifactId>commons-validator</artifactId>
<version>1.4.1</version>
</dependency>
build.gradle:
compile 'commons-validator:commons-validator:1.4.1'
The import:
import org.apache.commons.validator.routines.EmailValidator;
The code:
String email = "[email protected]";
boolean valid = EmailValidator.getInstance().isValid(email);
and to allow local addresses
boolean allowLocal = true;
boolean valid = EmailValidator.getInstance(allowLocal).isValid(email);
Solution 4
Late answer, but I think it is simple and worthy:
public boolean isValidEmailAddress(String email) {
String ePattern = "^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@((\\[[0-9]{1,3}\\.[0-9]{1,3}\\.[0-9]{1,3}\\.[0-9]{1,3}\\])|(([a-zA-Z\\-0-9]+\\.)+[a-zA-Z]{2,}))$";
java.util.regex.Pattern p = java.util.regex.Pattern.compile(ePattern);
java.util.regex.Matcher m = p.matcher(email);
return m.matches();
}
Test Cases:
For production purpose, Domain Name validations should be performed network-wise.
Solution 5
If you are trying to do a form validation received from the client, or just a bean validation - keep it simple. It's better to do a loose email validation rather than to do a strict one and reject some people, (e.g. when they are trying to register for your web service). With almost anything allowed in the username part of the email and so many new domains being added literally every month (e.g. .company, .entreprise, .estate), it's safer not to be restrictive:
Pattern pattern = Pattern.compile("^.+@.+\\..+$");
Matcher matcher = pattern.matcher(email);
Related videos on Youtube
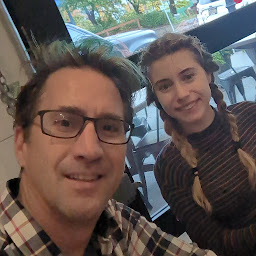
jon077
Updated on June 30, 2020Comments
-
jon077 almost 4 years
What are the good email address validation libraries for Java? Are there any alternatives to commons validator?
-
mpenkov over 11 yearsI'll just leave this here: davidcelis.com/blog/2012/09/06/…
-
james.garriss about 10 yearsCurrent URL for Commons: commons.apache.org/proper/commons-validator/apidocs/org/apache/…
-
Benny Bottema over 6 yearsYou shouldn't want to use libraries (or regexes) that don't comprehensively validate. Due to the complexity of valid email address, there is no middle-ground between no validation and comprehensive validation. Apache Commons' implementation is not comprehensive. I'm aware of only one library that is (email-rfc2822-validator), but it still works with huge regexes. A comprehensive lexer is what you really want. EmailValidator4J says it does the job, but I don't have experience with it.
-
Machavity almost 6 years@BennyBottema Instead of editing the question with commentary, please make a Meta post to discuss why this was closed if you still have questions.
-
-
duffymo over 15 yearsI agree with the extra bits you cited, but are those part of the Commons Validation project?
-
mist about 13 years1.4 SNAPSHOT also requires Jakarta ORO. Apache Commons Validator is not usable for me.
-
mist about 13 yearsFinally chose Dr.Vet. Cumpanasu Florin's solution: mkyong.com/regular-expressions/…
-
Matthew Flaschen almost 13 yearsNo, the Apache
EmailValidator
class does not send an email message for verification. -
Eduardo almost 13 yearsin the easiest and you probably already have it in your project to send emails
-
zillion1 over 12 yearsNote that InternetAddress.validate() considers user@[10.9.8.7] and user@localhost as valid email addresses - which they are according to the RFC. Though, depending on the use case (web form), you might want to treat them as invalid.
-
zillion1 over 12 yearsIf your use case is to validate a user's remote email address, this solution has a considerable flaw (similar to InternetAddress.validate()): EmailValidator considers user@[10.9.8.7] as a valid email addresses - which they are according to the RFC, but maybe not for user registration/contact form.
-
Matthew Flaschen over 12 years@zillion, that is documented in Apache COmmons: "This implementation is not guaranteed to catch all possible errors in an email address." And I said what you have to do to "ensure it's a real email". Addresses with local IPs could be valid in rare environments, though.
-
sarnold over 12 yearsRFC 2821 species (section 4.5.3.1) specifies a
local-part
length of 64 and adomain
length of 255. (They do say that longer is allowed by might be rejected by other software.) -
Nic Cottrell over 12 yearsAlso - I believe throwing and then catching exceptions is very inefficient
-
Nic Cottrell over 12 yearsI agree that the Apache Commons validator works well, but I find it to be quite slow - over 3ms per call.
-
mist over 12 yearsPerformance is not that important for me.
-
Jörg over 12 yearscurrent trunk SNAPSHOT (SVN REV 1227719 as of now) has no external dependencies like ORO anymore - you don't even need the whole validation module anymore - the four classes org.apache.commons.validator.routines.EmailValidator, InetAddressValidator, DomainValidator and RegexValidator are able to stand alone
-
TofuBeer about 12 yearsthe inefficiency shouldn't matter unless you are dealing with many invalid email addresses.
-
lacinato over 11 yearsI built upon Les Hazlewood's excellent class (which does have some bugs). (See my separate answer to this question.) Though I did maintain the java regex method, we use it just fine in a performance-critical environment. If all you're doing is parsing addresses, the performance may be an issue, but for most users I'd suspect it's just the beginning of whatever they're doing. My updates to the class did also fix a number of long-recursion issues.
-
Diego Plentz over 11 yearsnot only that is valid as @zillion1 said, but also things like bla@bla are considered valid. Really not the best solution.
-
gyorgyabraham over 11 years@NicholasTolleyCottrell This is Java, here we throw and catch exceptions, I don't really get your point
-
Nic Cottrell over 11 yearsMy point is that creating exceptions in Java is a relatively expensive process, whereas doing a validation returning a boolean would have much less overhead.
-
Martin Andersson over 11 yearsI suspect that InternetAddress constructor has been tampered with. Or my system has been tampered with. Or RFC822 has been tampered with. Or I could really use some sleep right now. But I just tried some code and the following five strings all pass as valid e-mail addresses if you pass them to the InternetAddress constructor, and "clearly", they are not valid. Here we go:
.
,.com
,com.
,abc
and123
. Also, adding leading or trailing white space do not invalidate the strings either. You be the judge! -
Arjan about 11 yearsWouldn't this also return true for
John Doe <[email protected]>
? This is a valid address to use in the email API, but might not be valid in other use cases. -
Robert Kang over 10 yearsThis is not really a good answer. The mentioned validator is way too generous on its rules. Email addresses like a@b should not be considered as valid.
-
Ajedi32 over 10 yearsYeah, so it seems that this method considers
asdf
and1234
to be valid email addresses... -
leojh over 10 yearsEmailValidator also gives you the option of excluding local email addresses so that the top level domain must be present, which was a requirement for me.
-
Sreedhar GS over 10 yearsIts even validating a single character as valid, which can't be accepted..!!
System.out.println(isValidEmailAddress("adafdad"));
-
Aaron Davidson over 10 yearsCorrectly returns false for me.
-
mlaccetti over 10 yearsThat's a pretty brutally simplistic validator that ignores most of the RFC rules along with IDNs. I'd steer clear of this for any production quality app.
-
user1050755 over 10 yearsEmailValidator is very, very, very simple. It is also limited to ASCII.
-
Piohen about 10 yearsApache Commons EmailValidator has one serious drawback: doesn't support IDN.
-
Alexander Burakevych about 10 years[email protected] will be not valid...
-
jwenting almost 10 years@RobertKang it is a valid email address according to the RFC, even if a non-existent one.
-
Josh Glover almost 10 yearsDon't roll your own regex-based validator for things covered by RFCs.
-
user2813274 over 9 yearsI am not sure the regex is the best way to do so, it's quite unreadable if you intend to follow the RFC to the letter
-
jmaculate over 9 yearsthis is a really good point, any reasonable app should have other measures to prevent this input from being exploited down the line anyway
-
Elad Tabak over 9 yearsOnly problem with apache commons is that it allows email addresses like "ab??$#@gmail.com". Although this address may be legit in terms of RFC, it's probably wrong input provided by the user.
-
Erik Kaplun over 9 yearsthis passes just fine but I don't think it should:
new InternetAddress(";...'[email protected],").validate
-
NimChimpsky over 9 years"cheese" is not a valid emai address, but it is according to this mechanism
-
Aaron Davidson over 9 yearsum, cheese fails properly when I run it. what the hell javax.mail library are you linking to???
-
dstibbe over 9 yearsPeople complaining about all kinds of non-existing, but valid email addresses being validated. It is a validator for the correctness of the email address, not whether it exists! After the validator a website should always employ an activation mechanism to check whether the address actually exists.
-
dldnh about 9 yearsreinventing the wheel is OK as long as you don't mind the occasional flat tire
-
Bake about 9 yearsThe "isValid(emailString)" method of commons-validator-1.4.1.jar library returns that email like "[email protected]" is invalid even it is valid email.
-
fiffy about 9 yearscommons-validator-1.4.1 reports
Leo Notenboom <[email protected]>
as invalid - althought it is accepted by common mailservers. -
brain storm about 9 yearsit validates even
a//[email protected]
, which it should not I suppose -
Alpha Huang almost 9 yearsHow about change it to "^.+@.+(\\.[^\\.]+)+$" to avoid a trailing dot?
-
David Balažic almost 9 yearsa//[email protected] is syntactically valid and all validators would accept it (unless they are broken).
-
Ismail Yavuz almost 9 yearsWhy InternetAddress is not recognized in jdk8.51?
-
Mikle Garin over 8 yearsIt also consider something like
[email protected]
as a valid email (tried with version 1.4.1). Or is it actually valid one? -
zOqvxf over 8 yearsIn Android Studio you can add compile 'commons-validator:commons-validator:1.4.1' into your app\build.gradle's dependencies {}
-
zOqvxf over 8 yearsAfter actually trying to build my project, it seems apache commons doesn't work with Android very well, hundreds of warnings and some errors, it didn't even compile. This is what I ended up using howtodoinjava.com/2014/11/11/java-regex-validate-email-address
-
Benny Bottema over 8 yearsHi, I copied it into GitHub for public the open source community. Now everybody can comment, document, and improve on the code. github.com/bbottema/email-rfc2822-validator. I used to use the older version by Les, but I had to remove it due to regex freezing bugs: leshazlewood.com/2006/11/06/emailaddress-java-class/…
-
Amit Mittal over 8 yearsSame problem with me as of Benjiko99. After adding the dependency, the project wont compile, says java.exe finished with non zero exit code 2.
-
Aksel Willgert over 8 yearsI think best option is to create new question to debug that crash
-
Christopher Schneider about 8 yearsThis answer isn't applicable anymore for obvious reasons. Remove TLD validation and it's probably acceptable if you want to accept non-English e-mail addresses.
-
Majora320 about 8 years@ismailyavuz You need to add the javax.mail library.
-
Matt over 7 yearsI was getting errors in Android Studio as well. I changed from 1.4.1 to 1.5.1 and it works!
-
j23 over 6 yearseven the latest 1.6.0 version treats valid trailing dots:[email protected]
-
HopeKing over 6 yearsNote: Use_the Emailvalidator in org.apache.commons.validator.routines since EmailValidator in org.apache.commons.validator is deprecated (I am using 1.6 commons Validator)
-
Benny Bottema over 6 yearsAlthough an alternative to Apache commons, its implementation is as rudimentary as most regex-based libraries. From the docs: "However, as this article discusses it is not necessarily practical to implement a 100% compliant email validator". The only regex based comprehensive validator I know is email-rfc2822-validator and otherwise EmailValidator4J seems promising.
-
Benny Bottema over 6 yearsAgree with @user2813274, you would want a proper lexer, not spaghetti regex.
-
Benny Bottema over 6 yearsThis is an outdated library and has been superseded twice, finally by email-rfc2822-validator. Although it still fits all modern needs, it is also still prone to hidden performance bugs (and doesn't support the limited changes by the newer RFC specs).
-
daticon over 6 yearsAgree the apache commons is better than the official java one..which didn't mark personsname@gmail as invalid.
-
Andrain over 6 yearsit is good but not for all the cases.
-
Dherik over 6 yearsWhy the downvote? It's the same class used by Hibernate Validator.
-
HendraWD about 6 yearsCheck commons.apache.org/proper/commons-validator/… for the newest version, and if it broken, you can just google it
-
HendraWD about 6 yearsCheck here for the newest version commons.apache.org/proper/commons-validator/…, or if the link is broken, you can just google it.