What is the correct input type for credit card numbers?
Solution 1
HTML
If you're trying to do this strictly with HTML, I believe the following is going to get you about as close as is currently possible:
<label for="ccn">Credit Card Number:</label>
<input id="ccn" type="tel" inputmode="numeric" pattern="[0-9\s]{13,19}" autocomplete="cc-number" maxlength="19" placeholder="xxxx xxxx xxxx xxxx">
-
inputmode
sets the keyboard type (in this case, numeric) -
pattern
enables validation (in this case, numbers and spaces with a length of between 13 and 19) and it helps with keyboard type for browsers that don't yet supportinputmode
-
autocomplete
tells browser what should be autocompleted (in this case, a credit card number) -
maxLength
prevents the user from entering more than the set number of characters (in this case, 19, to include numbers and spaces) -
placeholder
gives the user an example (formatting hint)
JavaScript
For a more robust solution, JavaScript is going to be required. And rather than roll your own solution, it'd probably be best to use a battle-tested library. Cleave.js (as you mentioned) is a popular choice.
Solution 2
There's an attribute inputmode
that's designed for that, it's not implemented yet (actually deprecated in HTML 5.2), but there's work done on it (FF/Chrome).
https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input
And see this discussion: https://github.com/whatwg/html/issues/3290
For now set the autocomplete attribute to the correct value: https://developers.google.com/web/updates/2015/06/checkout-faster-with-autofill
or implement a customized input with mask like you're using now.
Solution 3
I’ve seen using type="tel"
on many places, for the reasons you mention.
I would not recommend type="number"
as then you have to fiddle with in/decrement arrows etc. And from certain point of view it is not a “number” in terms of what we usualy do with numbers (maths), see this comment on CSS tricks forum.
Another trick how to force numeric keyboard is using pattern="[0-9]*"
. This should work on iOS only. To make it work on Android as well, you have to combine it with the type="tel"
.
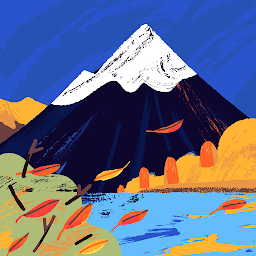
Micheal Friesen
Updated on January 16, 2020Comments
-
Micheal Friesen over 4 years
TLDR: Which input type can utilize the mobile numeric keyboards AND implement masking with spaces injected and a max limit for CVV and Credit Card inputs, such as: XXXX XXXX XXXX XXXX
When building forms, I have seen a varied degree of consistency surrounding the correct input type for credit cards. Here are my discovered pros and cons for each:
type=text
- Able to mask easily with various libraries such as cleave.js or set maxLength attribute
- Mobile users do not receive numeric-only keyboard, unless setting range to [0-9] (Only iOS users will get this experience, leaving Android users with full keyboard)
type=number
- Proper keyboard shown on iOS and Android but unwanted characters can be entered and no maxLength can be set. Min and Max do not limit users from inputting more than 16 characters but do provide error messages when over the max. *Note, this input type is basically ruled out due to leading 0's being deleted. (Unacceptable for CVV's)
type=tel
- Able to properly mask and is utilized all over the place, BUT may have unknown impacts on accessibility programs and autofillers. If anyone can provide clarification on the potential side effects of using this input type, that would be awesome!
These are all the types that came to mind. If anyone has any other recommendations, please let me know!
-
Micheal Friesen about 6 yearsSo you would recommend using type=tel and specifying the autofill attribute? Do you think there would be any side effects?
-
Micheal Friesen about 5 yearsI appreciate the response. I still think there should be a better standard for developers given how common this input field is.
-
Kout about 5 yearsI think there is, but not widely supported. It is the new attribute
inputmode
. In this case the mark-up would be following (simplified):<input type="text" inputmode="numeric">
. See the further info in the CSS tricks article. -
Micheal Friesen over 4 yearsThis is a great write up of probably the best you can do today. It just seems a bit strange that an input mode for something so commonly used is still subject to possible side effects due to the tel input type.
-
Kout almost 2 yearsIs it really deprecated? It is a living standard now. I think we can use it now at 2022. html.spec.whatwg.org/multipage/interaction.html#attr-inputmode