What is the difference between a field and a property?
Solution 1
Properties expose fields. Fields should (almost always) be kept private to a class and accessed via get and set properties. Properties provide a level of abstraction allowing you to change the fields while not affecting the external way they are accessed by the things that use your class.
public class MyClass
{
// this is a field. It is private to your class and stores the actual data.
private string _myField;
// this is a property. When accessed it uses the underlying field,
// but only exposes the contract, which will not be affected by the underlying field
public string MyProperty
{
get
{
return _myField;
}
set
{
_myField = value;
}
}
// This is an AutoProperty (C# 3.0 and higher) - which is a shorthand syntax
// used to generate a private field for you
public int AnotherProperty { get; set; }
}
@Kent points out that Properties are not required to encapsulate fields, they could do a calculation on other fields, or serve other purposes.
@GSS points out that you can also do other logic, such as validation, when a property is accessed, another useful feature.
Solution 2
Object orientated programming principles say that, the internal workings of a class should be hidden from the outside world. If you expose a field you're in essence exposing the internal implementation of the class. Therefore we wrap fields with Properties (or methods in Java's case) to give us the ability to change the implementation without breaking code depending on us. Seeing as we can put logic in the Property also allows us to perform validation logic etc if we need it. C# 3 has the possibly confusing notion of autoproperties. This allows us to simply define the Property and the C#3 compiler will generate the private field for us.
public class Person
{
private string _name;
public string Name
{
get
{
return _name;
}
set
{
_name = value;
}
}
public int Age{get;set;} //AutoProperty generates private field for us
}
Solution 3
An important difference is that interfaces can have properties but not fields. This, to me, underlines that properties should be used to define a class's public interface while fields are meant to be used in the private, internal workings of a class. As a rule I rarely create public fields and similarly I rarely create non-public properties.
Solution 4
I'll give you a couple examples of using properties that might get the gears turning:
- Lazy Initialization: If you have a property of an object that's expensive to load, but isn't accessed all that much in normal runs of the code, you can delay its loading via the property. That way, it's just sitting there, but the first time another module tries to call that property, it checks if the underlying field is null - if it is, it goes ahead and loads it, unknown to the calling module. This can greatly speed up object initialization.
- Dirty Tracking: Which I actually learned about from my own question here on StackOverflow. When I have a lot of objects which values might have changed during a run, I can use the property to track if they need to be saved back to the database or not. If not a single property of an object has changed, the IsDirty flag won't get tripped, and therefore the saving functionality will skip over it when deciding what needs to get back to the database.
Solution 5
Using Properties, you can raise an event, when the value of the property is changed (aka. PropertyChangedEvent) or before the value is changed to support cancellation.
This is not possible with (direct access to) fields.
public class Person {
private string _name;
public event EventHandler NameChanging;
public event EventHandler NameChanged;
public string Name{
get
{
return _name;
}
set
{
OnNameChanging();
_name = value;
OnNameChanged();
}
}
private void OnNameChanging(){
NameChanging?.Invoke(this,EventArgs.Empty);
}
private void OnNameChanged(){
NameChanged?.Invoke(this,EventArgs.Empty);
}
}
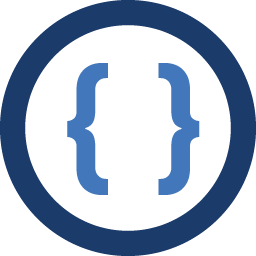
Admin
Updated on February 15, 2022Comments
-
Admin about 2 years
In C#, what makes a field different from a property, and when should a field be used instead of a property?
-
Sekhat over 15 yearssince when? lock your backing field within the property and it's the equivilant
-
Jonathan C Dickinson over 15 yearsProperties are methods, and are not inlined by any CIL JIT today. If you are going to use thread primitives like Interlocked you need to have fields. Check your sources. Admittedly 'locking' was the wrong word to use.
-
frankgut almost 15 yearsProperties should never have side effects. Even the debugger assumes it can evaluate them safely.
-
Brian Rasmussen almost 15 years@Strilanc: I agree completely, however, that is not always the case. As for the debugger, there are many problems with FuncEval if that is what you're talking about.
-
sites over 11 yearsA question about dirty tracking: what if I could change the field directly– I don't know if that can be done, I could say: "the object does not need to be saved if not a single FIELD of an object has changed" thus dirty tracking would not be a difference, am I missing something?
-
supercat about 11 years@juanpastas: The advantage of properties with regard to dirty tracking is that if property setters will set a "dirty" flag, then in the scenario where the flag isn't set code won't have to inspect the values of any properties to see if they might have changed. By contrast, if an object exposes its attributes as fields, then the contents of all fields must be compared against the previous value (which not only adds time to do the comparison, but also means the code must have the previous value).
-
Priidu Neemre over 10 years+1 for mentioning autoproperties - I think this is something many of the answers here (and elsewhere) have forgotten to bring in. Without this explanation, it can still be pretty hard to grasp what
public int myVar { get; set; }
really stands for (and I presume that it's the reason for at least 50% of the hits this question gets). -
DavidRR over 10 yearsSince C# 3.0, the pattern described here is conveniently supported by a feature called Auto-Implemented Properties.
-
Chucky almost 10 years"while not affecting the external way they are accessed by the things that use your class." forgive me if I'm incorrectly understanding, then, but why the need for access modifiers in front of properties at all, if the field behind it seems to handle this? i.e. why make a property anything other than public?
-
Nyra almost 10 years+1 also for mentioning auto, and mentioning how it works ("AutoProperty generates private field for us") This was the answer I've been looking for to a question I had. When researching I didn't see on MSDN's page about them any indication that a private field was created and was causing confusion. I guess that's what this means? "Attributes are permitted on auto-implemented properties but obviously not on the backing fields since those are not accessible from your source code. If you must use an attribute on the backing field of a property, just create a regular property." but wasn't sure.
-
AdamMc331 over 9 years@Chucky I asked a similar question in Java, that might apply here. Question
-
David Ferenczy Rogožan about 9 yearsThese are interesting facts, but you're missing the point of fields and properties philosophy.
-
Mark Lakata almost 9 yearsI think one of the advantages in C# with Properties as they have the same API as fields, so clients of the class don't really care if they are accessing a property or a field. (This is NOT true in C++ for example).. In prototyping, I think it is reasonable to start with public fields, and then migrate to properties as needed. There is a performance and memory hit with properties, and there is extra typing. They are not free. But, if you change your mind, you won't need to refactor any dependent code.
-
Sarath Subramanian almost 9 yearsWhat do u mean by PHILISOPHY? @Dawid Ferenczy
-
Veverke almost 9 yearsTo add to the "completeness" of the dicussion, we are differentiating a property from a field, saying that a property is an abstraction of the former (but not necessarily, as Kent notes). In our context field and attribute are interchangeable ?
-
David Ferenczy Rogožan almost 9 yearsSee for example marked answer. But you noticed, that you're just providing an usage examples, since difference between fields and properties was already described, so forgot my comment, please :)
-
David Ferenczy Rogožan almost 9 yearsI have read it, but you didn't read my previous comment obviously: "But you noticed, that you're just providing an usage examples, since difference between fields and properties was already described, so forgot my comment, please :)".
-
Suamere over 8 yearsYour answer was right before the edits and oddly-upvoted incorrect comments. A property should always encapsulate one or more fields, and should never do any heavy lifting or validation. If you need a property such a UserName or Password to have validation, change their type from strings to Value Objects. There is an unspoken contract between a class-creator and the consumer. Fields hold state, Properties expose state using one or more fields, Voids change state (heavy lifting), and Functions perform queries(heavy lifting).This is not stone, just loose expectations.
-
Suamere over 8 years@KentBoogaart - Without encapsulating fields, a property would have one of a few possibilities:
get { return "hello"; }
. Or Functions:get { return someFunction(); }
. Why not just make constants and functions? Just so consumers don't need to supply()
? A consumer should trust that calling a()
means there may be taxing work, and calling a property means there will be zero taxing work. And when would a setter not change the state of the object (therefore changing a field). I realize you CAN do these things, but certainly shouldn't be discussed in public. -
sara over 8 yearsNote that the given example doesn't encapsulate squat. This property gives 100 % full access to the private field, so this isn't object-oriented at all. You might as well have a public field in this case. Granted, it helps (marginally) to refactor code in the future, but any IDE worth it's mettle can transform a field to a property with a few keystrokes. The answer might be technically correct about how properties work, but it does not give a good "OOP explanation" to their uses.
-
Gobe about 8 years@kai I agree that the answer over-simplified things and is not showing all the power of an auto-property, however I disagree that this is not object-oriented. You may want to check the difference between fields and properties. Fields cannot be virtual, and
virtual
itself is part of object-oriented programming. -
sara about 8 yearsGranted, there are some functional difference. I would not call
virtual
OOP per se though. It's a tool that enables polymorphism, which is one of the key tools that ENABLES OOP. It's not OOP in and of itself though, and there is nothing inherently OOP about a public autoproperty. I wouldn't count stuff such as reflection or databinding OOP related either. Normally I wouldn't be so pedantic about it, but the answer specifically mentioned OO principles as the driving force behind the code example, and I don't agree with that. -
coloboxp over 7 yearsThose is a good ones It also allows you to trigger methods (as events), or logging when the value is set or readed.
-
Admin over 7 yearsI took a long time to find this. This is a MVVM. Thank you ! :)
-
Admin over 7 yearsYeah my bad, sorry!
-
jpaugh over 7 years@Suamere Why shouldn't a setter do validation?
-
Suamere over 7 years@jpaugh If I am a class consumer, I follow contracts set by the class creator. If a property is
string
, my contract is: assign any chars up to ~2bil length. If a property isDateTime
, my contract is: assign any numbers within the limits of DateTime, which I can look up. If the creator adds constraints to the setters, those constraints are not communicated. But if, instead, the creator changes the type fromstring
toSurname
, then their new Surname class communicates the constraints, and the propertypublic Surname LastName
doesn't have setter validation. Also,Surname
is reusable. -
Suamere over 7 yearsAnd since
Surname
, in my example, is reusable, you don't need to worry about later on copy/pasting those validations in a property setter to other places in code. Nor wondering if the validation of a Surname is in multiple places if you ever make changes to the business rules for Surnames. Check out the link I posted about Value Objects -
jpaugh about 7 years@Suamere Putting validation logic into the setter provides a different contract: that the object can never get into an invalid state wrt. the properties which are validated. When all of the accessors are validated (as appropriate) and no fields are exposed without such validation, this contract, which I'll call "Setting never causes failure of the object," is often more useful than, "setting never fails." Is any such contract really communicated without reading the source? No, not really. That's rather part of the point of properties; only the behavior of fields have such transparency.
-
Suamere about 7 years@jpaugh You are on the right track to where I stand. You say no, contracts are never really communicated without reading the source. But contracts are easily communicated and validation easily implemented if you use Value Objects as I mentioned, and so we're back to square one on where I stand.
-
jpaugh about 7 years@Suamere You don't say? Haskell has built-in support for such "contract-only type wrappers" (and calls them
newtype
s), but I hadn't considered implementing them myself in an OO language. (Thanks!) In Haskell, they don't add any run-time overhead, which is nice: "after the type is checked at compile time, at run time the {newtype wrapper} can be treated essentially the same, without the overhead or indirection normally associated..." -
HeartWare over 6 yearsProperties can't be used as OUT or REF parameters, so changing a field into a property could lead to compile errors down the line. If the value was implemented as a property from the beginning, then it would never have been used as OUT or REF (VAR in Pascal/Delphi) parameters, and any change you make in the getter/setter would be transparent to the usage.
-
shivesh suman over 6 yearsThere is a lot of discussion on this question and on the information being shared in the answer and the contents of comments. For this question it may be a good idea to actually consult the documentation: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…
-
Steve Bauman almost 6 yearsThis is an awesome answer, really helped me understand this.
-
Oddmar Dam almost 5 yearsHello and welcome to StackOverflow. Please take some time to read the help page, especially the sections named How to Answer. You might also want to learn about minimal reproducible example.
-
Ted Mucuzany almost 5 yearsHello and thank you! Is it something wrong with my answer? I've read all the previous ones and found them too wordy. My one is the essence of the difference between fields and properties in c#, I believe. It is as short as possible, but still explains the thing.
-
Oddmar Dam almost 5 yearsHi @Ted. Iw'e worked on c# for about 12 years and have never thought about what the side effects are :) Would love to hear about them. But i agree, the answer is short and precise. Just needs a bit more detail :)
-
Ted Mucuzany almost 5 years@Oddmar Dam, I enumerated them in brackets. An example could be this (dotnetfiddle.net/SFWC1d)
-
Denny Jacob about 4 years@sara "This property gives 100% full access to the private field, so this isn't object-oriented at all." You miss the point. Encapsulation is that a consumer of the object will not know how the value is resolved. In the case of property, field is one of the possibilities. It could be a cookie instead of a field.
-
Gary almost 4 years"A property is a member that provides a flexible mechanism to read, write, or compute the value of a private field." This makes the most sense.
-
Eagle_Eye over 3 yearsCurious what that rare scenarios could be!
-
stenci about 3 yearsThis answer and the answers below are correct. The 2 most voted posts are not answering the question. The question is "what is the difference...", but the most voted answers respond with "instead of telling you what is the difference I'm telling you how you should work"
-
stenci about 3 yearsThis does not address the question. The question is "what is the difference...", this post says "instead of telling you what is the difference I'm telling you how you should work"
-
stenci about 3 yearsThis does not address the question. The question is "what is the difference...", this post says "instead of telling you what is the difference I'm telling you how you should work"
-
OCDev over 2 years@sara is right. The way properties and auto properties are used here don't do anything to benefit the principle of OOP stated here. You might as well use a field and then change the field to a property later when you want to add special functionality for getting and setting. Starting off by making everything have unused getters and setters is a popular practice in the C# world, but it violates principles that are against over-engineering and it breaks encapsulation in disguise. Popular isn't always correct.