What is the difference between a variable, object, and reference?
Solution 1
(Just to be clear, the explanation I'm giving here is specific to Java and C#. Don't assume it applies to other languages, although bits of it may.)
I like to use an analogy of telling someone where I live. I might write my address on a piece of paper:
- A variable is like a piece of paper. It holds a value, but it isn't the value in itself. You can cross out whatever's there and write something else instead.
- The address that I write on the piece of paper is like a reference. It isn't my house, but it's a way of navigating to my house.
- My house itself is like an object. I can give out multiple references to the same object, but there's only one object.
Does that help?
The difference between a value type and a reference type is what gets written on the piece of paper. For example, here:
int x = 12;
is like having a piece of paper with the number 12 written on it directly. Whereas:
Dog myDog = new Dog();
doesn't write the Dog object contents itself on the piece of paper - it creates a new Dog
, and then writes a reference to the dog on that paper.
In non-analogy terms:
- A variable represents a storage location in memory. It has a name by which you can refer to it at compile time, and at execution time it has a value, which will always be compatible with its compile-time type. (For example, if you've got a
Button
variable, the value will always be a reference to an object of typeButton
or some subclass - or thenull
reference.) - An object is a sort of separate entity. Importantly, the value of a variable or any expression is never an object, only a reference. An object effectively consists of:
- Fields (the state)
- A type reference (can never change through the lifetime of the object)
- A monitor (for synchronization)
- A reference is a value used to access an object - e.g. to call methods on it, access fields etc. You typically navigate the reference with the
.
operator. For example, iffoo
is aPerson
variable,foo.getAddress().getLength()
would take the value offoo
(a reference) and callgetAddress()
on the object that that reference refers to. The result might be aString
reference... we then callgetLength()
on the object that that reference refers to.
Solution 2
I often use the following analogy when explaining these concepts.
Imagine that an object is a balloon. A variable is a person. Every person is either in the value type team or in the reference type team. And they all play a little game with the following rules:
Rules for value types:
- You hold in your arms a balloon filled with air. (Value type variables store the object.)
- You must always be holding exactly one balloon. (Value types are not nullable.)
- When someone else wants your balloon, they can blow up their own identical one, and hold that in their arms. (In value types, the object is copied.)
- Two people can't hold the same balloon. (Value types are not shared.)
- If you want to hold a different balloon, you have to pop the one you're already holding and grab another. (A value type object is destroyed when replaced.)
Rules for reference types:
- You may hold a piece of string that leads to a balloon filled with helium. (Reference type variables store a reference to the object.)
- You are allowed to hold one piece of string, or no piece of string at all. (Reference type variables are nullable.)
- When someone else wants your balloon, they can get their own piece of string and tie it to the same balloon as you have. (In reference types, the reference is copied.)
- Multiple people can hold pieces of string that all lead to the same balloon. (Reference type objects can be shared.)
- As long as there is at least one person still holding the string to a particular balloon, the balloon is safe. (A reference type object is alive as long as it is reachable.)
- For any particular balloon, if everyone eventually lets go of it, then that balloon flies away and nobody can reach it anymore. (A reference type object may become unreachable at some point.)
- At some later point before the game ends, a lost balloon may pop by itself due to atmospheric pressure. (Unreachable objects are eligible for garbage collection, which is non-deterministic.)
Solution 3
You can think of it like a answering questions.
An object is a what...
It's like any physical thing in the world, a "thing" which is recognizable by itself and has significant properties that distinguishes from other "thing".
Like you know a dog is a dog because it barks, move its tail and go after a ball if you throw it.
A variable is a which...
Like if you watch your own hands. Each one is a hand itself. They have fingers, nails and bones within the skin but you know one is your left hand and the other the right one.
That is to say, you can have two "things" of the same type/kind but every one could be different in it's own way, can have different values.
A reference is a where...
If you look at two houses in a street, although they're have their own facade, you can get to each one by their one unique address, meaning, if you're far away like three blocks far or in another country, you could tell the address of the house cause they'll still be there where you left them, even if you cannot point them directly.
Now for programming's sake, examples in a C++ way
class Person{...}
Person Ana = new Person(); //An object is an instance of a class(normally)
That is to say, Ana is a person, but she has unique properties that distinguishes her from another person.
&Ana //This is a reference to Ana, that is to say, a "where" does the variable
//"Ana" is stored, wether or not you know it's value(s)
Ana
itself is the variable for storing the properties of the person named "Ana"
Solution 4
Jon's answer is great for approaching it from analogy. If a more concrete wording is useful for you, I can pitch in.
Let's start with a variable. A variable is a [named] thing which contains a value. For instance, int x = 3
defines a variable named x, which contains the integer 3. If I then follow it up with an assignment, x=4
, x now contains the integer 4. The key thing is that we didn't replace the variable. We don't have a new "variable x whose value is now 4," we merely replaced the value of x with a new value.
Now let's move to objects. Objects are useful because often you need one "thing" to be referenced from many places. For example, if you have a document open in an editor and want to send it to the printer, it'd be nice to only have one document, referenced both by the editor and the printer. That'd save you having to copy it more times than you might want.
However, because you don't want to copy it more than once, we can't just put an object in a variable. Variables hold onto a value, so if two variables held onto an object, they'd have to make two copies, one for each variable. References are the go-between that resolves this. References are small, easily copied values which can be stored in variables.
So, in code, when you type Dog dog = new Dog()
, the new operator creates a new Dog Object, and returns a Reference to that object, so that it can be assigned to a variable. The assignment then gives dog
the value of a Reference to your newly created Object.
Solution 5
new Dog() will instantiate an object Dog ie) it will create a memory for the object. You need to access the variable to manipulate some operations. For that you need an reference that is Dog myDog. If you try to print the object it will print an non readable value which is nothing but the address.
myDog -------> new Dog().
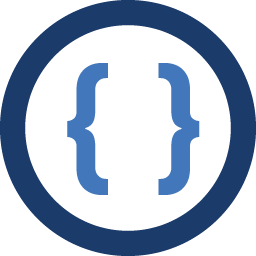
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Exactly what are the differences between variables, objects, and references?
For example: they all point to some type, and they must all hold values (unless of course you have the temporary null-able type), but precisely how are their functions and implementations different from each other?
Example:
Dog myDog = new Dog(); //variable myDog that holds a reference to object Dog int x = 12; //variable x that hold a value of 12
They have the same concepts, but how are they different?
-
Mdev over 8 yearsNot sure what the difference between an object/variable is according to these definitions.
-
Sergey Shcherbakov over 8 years12 items to understand the difference between 3 simple notions?
-
Theodoros Chatzigiannakis over 8 years@Smollet Well, yes.Since the OP asked, apparently it wasn't that simple for him/her (and probably for other people who end up here). I'm sure one can give a simpler analogy in a shorter answer, but I peronally found all the above points important enough to include.
-
Intellectual Gymnastics Lover about 3 yearsWhere is the asterisk
*
in your C++ examplePerson Ana = new Person();
? It should bePerson* Ana = new Person();
, right?