What is the difference between header file and namespace?
Solution 1
Header files are actual files - stored in the file system, referenced by file name, and #include
'd in other files (at least, in C/C++ or other languages using the M4 macro preprocessor). Header files typically group pieces of code that are all interdependent parts of the same specific item together. For instance, a game might have a header file for all of its graphics rendering.
Namespaces, on the other hand, are an element of the programming language - they don't exist as a file system object, but rather as a designation within code telling the compiler that certain things are within that namespace. Namespaces typically group interfaces (functions, classes/structs, types) of similar (but not necessarily interdependent) items. For instance, the std
namespace in C++ contains all of the Standard Library functions and classes.
Solution 2
To know what is Header file, you need to know the meaning of "declaration".
To put it in simple words, in C/C++, compilation happens in per-source manner. If I have a A.cpp and inside I make use of a function foo(), which will be defined somewhere else, I need to tell the compiler that: "Hey, I am using foo(), although you cannot see it defined anywhere in my source, don't worry, it is defined in another source". They way to tell compiler about this is by "declaring" foo() in A.cpp.
If I am the author of foo(), everyone that use foo() need to write down the declaration void foo(); in their source file. It will be a lot of duplicated and meaningless work. And it is so difficult for me to tell the guy that "use" foo() to have a correct declaration. Therefore as the author of foo(), I write down a file, containing the declaration for using foo(), and distribute it so that people can just "import" the file content to their source. The file I am distributing is Header file. The action of import is #include in C/C++. Yes, #include is nothing but inserting the content of the included file to the spot of #include.
Namespace is another story. To make it short, you can think of it is "real" name of function/class etc. for example, if I make
namespace FOO {
class Bar { }
}
The class is not really named Bar, it's "real" name is in fact FOO::Bar.
C++ provides some way to save you typing the long real name, by "using".
Solution 3
A header file is a file that is intended to be included by source files. They typically contain declarations of certain classes and functions.
A namespace enables code to categorize identifiers. That is, classes, functions, etc. can be placed inside a namespace, keeping those separate from other classes that are unrelated. For example, in C++ everything from the standard library is in the std
namespace.
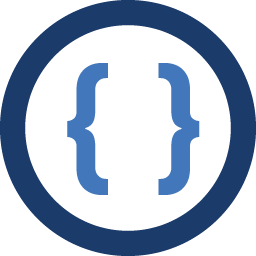
Admin
Updated on June 09, 2022Comments
-
Admin about 2 years
I want to know the exact difference between Header file (as in MyHeader.hpp) and a
namespace
inc++
? -
Admin almost 14 yearsHeaderfile contains Prototype,declarations.
-
GManNickG almost 14 years@Vish: Are you telling me or asking me? Strictly speaking, there's no such thing as a prototype; that's just a declaration.
-
spruceb almost 8 yearsYour answer contains some useful information, and would probably have been better received if you made put more effort into writing readable english, and gave more details. Hopefully you have a good experience on StackOverflow!
-
Jayraj Srikriti Naidu almost 8 yearsThanks! I have done some edits. Next time I will improve over them.