What is the difference between on("click",function()) and onclick="function();"?
Solution 1
<input type="button" onclick="alert('hello world');">
This is the way how inline events are handled. The main reason this is a bad idea is to clearly define the separation of concerns.
HTML - Structure of your page
JS - Your page functionality
This will cause less maintenance issues in the long run and when the system scales in size.
What happens if you have 100 buttons on your page and you want to remove the click event or change it for all of them. It would definitely be a nightmare. Also you can only define a single event if you bind it inline.
By moving out to a separate file you have a lot of flexibility and you can just make a small change that will affect all the elements on the page.
So the 2nd approach is always better. and the way to go.
By defining the events like below
$('#clickme').on("click",function(){alert('hello world');});
you HTML
looks clean sans of any functionality and removes the tight coupling.
In the cases you have a dynamically added, it is true inline events always work but there is a concept called Event Delegation . You attach the event to a parent container that is always present on the page and bind the event to it. When the event occurs at a later time on the element , the event bubbles to the parent which handles the event for you.
For such cases you bind the events using .on
passing in a selector
$(document).on('click', '#clickme', function() {
Keep in mind that binding multiple events to a document is a bad idea. You can always use the closestStaticAncestor
to bind the events.
Solution 2
The first approach only lets you register one click listener whereas the second approach lets you register as many as you want.
If you want clicks on dynamically added elements to be heard as well, you should use .on()
. Here's some code that demonstrates this (jsfiddle):
HTML
<div id="mainDiv">
<span class="span1">hello</span>
</div>
JS
$(document).on("click", ".span1", function () {
$( "#mainDiv" ).append("<span class=\"span1\">hello</span>");
});
$(".span1").click( function () {
console.log( "first listener" );
});
$(".span1").click( function () {
console.log( "second listener" );
});
Notice that first listener
and second listener
is only printed when clicking on the first hello, whereas a new span
gets added when clicking on any of the spans.
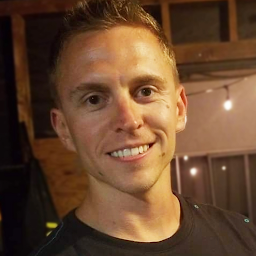
oliver_siegel
Updated on July 10, 2022Comments
-
oliver_siegel almost 2 years
So if I want something to happen when I click a button I can either do:
<!-- EXAMPLE A--> <input type="button" onclick="alert('hello world');">
or I can do
<!-- EXAMPLE B--> <input type="button" id="clickme"> <script> $('#clickme').on("click",function(){alert('hello world');}); </script>
Or of course any variations (on
change
, onhover
) and shortcuts (.click()
.change()
) are possible...Besides the fact that A is shorter, what are the differences? Which is better and why?
I noticed, that when I use
.html()
to dynamically add an element to the site (like a button) B doesn't work for this newly created button, and I need to use A...Any insights would be helpful!
-
go-oleg almost 11 years@SamuelLiew: Haha. I've heard of answer posters downvoting other answers but not the question asker! Oh well.
-
Cory Danielson almost 11 yearsYou're "guessing" that the only question is related to delegates, yet there are 2 questions above his delegates statement which are highlighted in bold. Read the question and answer it completely instead of fishing for reputation by trying to provide the quickest answers to the easiest questions you can find.
-
oliver_siegel almost 11 yearsHey I only downvoted the one answer that had nothing to do with my question. All others I upvoted!
-
Cory Danielson almost 11 yearsA full well-written answer - bravo.
-
Vishal Kumar Sahu about 7 yearsYou are overwriting the attribute... Try
$('#JQueryAttrClick').attr('onClick'," alert('2');alert('3');" );
But it helped me in my context. Thanks...