What is the fastest way of converting an array of floats to string?
Solution 1
I would go for the most readable string.Join
which also should have sufficient performance in most cases. Unless there is a real issue, I would not run my own:
float[] values = { 1.0f, 2.0f, 3.0f };
string s = string.Join(" ", values);
It might be that I misread your question, so in case you want an enumeration of string
go with the other answers.
Solution 2
To be more explicit, call float.ToString()
manually and then string.Join()
to separate each result with a space:
var array = new float[] { 0.1, 1.1, 1.0, 0.2 };
string result = String.Join(" ", array.Select(f => f.ToString(CultureInfo.CurrentCulture));
btw,
in .NET 2.0/3.0/3.5 there only single String.Join(string, string[])
but in .NET 4.0 there is also String.Join<T>(string, IEnumerable<T>)
@0xA3 uses method from .NET 4.0. Mine too. So for earlier versions use array.Select(..).ToArray()
Solution 3
float[] arr = { 1.0f, 2.1f };
var str = arr.Select(x => x.ToString()).ToArray();
or use rray.ConvertAll
public static string FloatFToString(float f)
{
return f.ToString();
}
float[] a = { 1.0f, 2.1f };
var res = Array.ConvertAll(a, new Converter<float, string>(FloatFToString));
Solution 4
You can do it like this:
var floatsAsString = yourFloatArray.Select(f => f.ToString(CultureInfo.CurrentCulture));
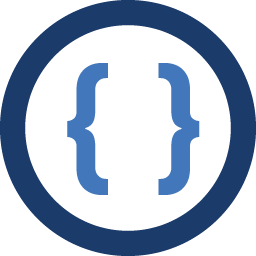
Admin
Updated on July 21, 2022Comments
-
Admin almost 2 years
What is the fastest way of converting an array of floats into string in C#?
If my array contains this
{ 0.1, 1.1, 1.0, 0.2 }
Then I want each entry to converted to a string with value separated by a white space, i.e.
"0.1 1.1 1.0 0.2"
-
abatishchev over 13 yearsProbably, it will just call
float.ToString()
for each element in the array -
Admin over 13 yearsIsn't compiling. Says "Join" has some invalid arguments.
-
Dirk Vollmar over 13 years@abatishchev: Yes, it will, and then it uses a string builder to concatenate the elements. If you need specific formatting then your answer offers all possibilities.
-
Dirk Vollmar over 13 years@Wajih: Sorry, I forgot to check it, but my solution only works with .NET 4.0.
-
Admin over 13 yearsDude, you're the man, thanks indeed!!!
-
C.J. over 13 yearsthis answer is readable. The 'checked' answer is not.
-
abatishchev over 13 yearsUsing
String.Concat
andString.Format
definitely is very inefficient -
LukeH over 13 years@Wajih: If you need it to work in older versions of .NET then just do
string s = string.Join(" ", values.Select(f => f.ToString()).ToArray())
-
iburlakov over 13 years@abatishchev So, what is better way?
-
Dirk Vollmar over 13 yearsThis seems to be the most complete answer.
-
abatishchev over 13 yearsMine or @0xA3's way, I guess. Because
String.Join
usesStringBuilder
internally that's much more efficient because of strings immutability in .NET. -
iburlakov over 13 years@abatishchev Thanks for info, i didn't know that String.Join uses StringBuilder internally.
-
abatishchev over 13 yearsYou can investigate such things using RedGate .NET Reflector
-
abatishchev over 13 yearsAlso as far as
Array
has not its own methodAggregate
there is no need to callAsEnumerable
becauseArray
is alreadyIEnumerable