What is the overhead cost of an empty vector?
Solution 1
As for the question as asked: It depends on the implementation. With MSVC 7.1 this:
std:: cout << sizeof(std::vector<int>) << std::endl;
gives me 16 (bytes). (3 pointers: begin, end, and end of capacity, plus an allocator)
However it should be noted that the pointer-to-vector gives it a larger overhead:
- in both time and space in the non-empty case
- in complexity in all cases.
Solution 2
It's completely implementation-dependent and you should neither assume nor rely on the details. For what it's worth it's 20-bytes using VC.
Solution 3
std::vector v;
takes up sizeof(v)
space. It might vary by implementation, so run it and find out how much it takes for you.
Solution 4
In Visual Studio Community 2017 (Version 15.2), running this code:
#include <iostream>
#include <vector>
using namespace std;
void main()
{
vector<float> test;
vector<float>* test2 = &test;
cout << sizeof(test) << "\n";
cout << sizeof(test2) << "\n";
cout << "\n";
system("pause");
}
Running in 32 bit (x86), I get 16 bytes for the vector and 4 bytes for the vector pointer.
Running in 64 bit (x64), I get 32 bytes for the vector and 8 bytes for the vector pointer.
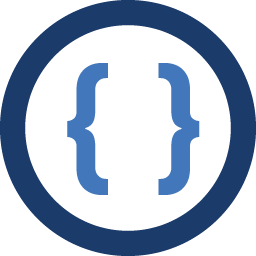
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
What is the memory overhead of having an empty vector vs having a pointer to a vector?
Option A:
std::vector<int> v;
Option B:
std::vector<int> *v = NULL;
I believe that option B takes 1 32 bit pointer (assuming 32 bit here) How much memory does the empty 'v' take up?