What tools are available to auto-produce documentation for a REST API written in Flask?
Solution 1
I would recommend you Sphinx, you add your documentation as __doc__
and the autodoc
module of Sphinx will generate the docs for you (docs.python.org also uses Sphinx). The markup is reStructuredText, similiar to Markdown (if you prefer Markdown, you can use pdoc).
e.g.:
@app.route('/download/<int:id>')
def download_id(id):
'''This downloads a certain image specified by *id*'''
return ...
Solution 2
I really like Swagger because it allows to generate an API documentation by just adding a few decorators and comments into your code. There is a Flask Swagger available.
from flask import Flask
from flask.ext.restful import Api
from flask_restful_swagger import swagger
app = Flask(__name__)
api = swagger.docs(Api(app), apiVersion='1', api_spec_url="/api/v1/spec")
class Unicorn(Resource):
"Describing unicorns"
@swagger.operation(
notes='some really good notes'
)
def get(self, todo_id):
...
Then you can see your methods and notes in an html interface just by visiting /api/v1/spec (it serves needed static automatically). You can also just get all your API description in JSON and parse it otherwise.
Solution 3
There is a Flask extension: flask-autodoc for auto documentation specially parsing endpoint route rule. You can add doc
decorator to specify which APIs you want to doc:
@app.route('/doc')
@auto.doc()
def documentation():
'''
return API documentation page
'''
return auto.html()
@app.route('/')
@auto.doc()
def welcome():
'''
Welcome API
'''
commit_hash = subprocess.check_output(["git", "rev-parse", "HEAD"])
commit_msg = subprocess.check_output(["git", "log", "-1", "--format=%s"])
date_time = subprocess.check_output(["git", "log", "-1", "--format=%cd"])
return "Welcome to VM Service Server. <br/>" \
"The last commit: %s<br/>Date: %s, <br>Hash: %s" % \
(commit_msg, date_time, commit_hash), 200
The simple html documentation page is like this:
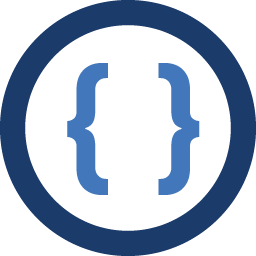
Admin
Updated on January 11, 2020Comments
-
Admin over 4 years
I'm looking for a quick way to auto produce REST API docs from a Flask REST API I've written. Does anyone know of tools that can do this and how I would markup the code?