when I import firebase using import * as firebase from 'firebase';?
Solution 1
You should update the imports & code this way to make it work out.
import firebase from 'firebase/compat/app';
import 'firebase/compat/auth';
import 'firebase/compat/firestore';
const firebaseConfig = { // Have the firebase config here
apiKey: "",
authDomain: "",
projectId: "",
storageBucket: "",
messagingSenderId: "",
appId: "",
measurementId: ""
};
// Use this to initialize the firebase App
const firebaseApp = firebase.initializeApp(firebaseConfig);
// Use these for db & auth
const db = firebaseApp.firestore();
const auth = firebase.auth();
export { auth, db };
Solution 2
In the docs of firebase
npm package they say:
If you are using native ES6 module with --experimental-modules flag, you should do:
// This import loads the firebase namespace. import firebase from 'firebase/app'; // These imports load individual services into the firebase namespace. import 'firebase/auth'; import 'firebase/database';
So try replacing
import * as firebase from 'firebase'
with
import firebase from 'firebase/app'
You may also consider using firebase wrapper build for react-native https://github.com/invertase/react-native-firebase. It will require from you an extra setup described in the docs, but works better.
Solution 3
Try:
npm install --save firebase
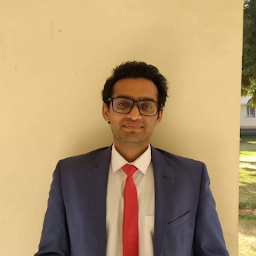
Chirag Gupta
Updated on June 10, 2022Comments
-
Chirag Gupta about 2 years
shows me this error?
Unable to resolve "firebase" from "App.js" Failed building JavaScript bundle.
I have tried looking for answers but these were for previous verisons for firebase I also tried adding this import too but didn't solved my problem.
import firebase from '@firebase/app'
}, "dependencies": { "expo": "^33.0.0", "firebase": "^6.3.0", "native-base": "^2.12.1", "react": "16.8.3", "react-dom": "^16.8.6", "react-native": "https://github.com/expo/react-native/archive/sdk- 33.0.0.tar.gz", "react-native-gesture-handler": "^1.3.0", "react-native-web": "^0.11.4", "react-navigation": "^3.11.1" },
can someone help
-
Chirag Gupta almost 5 yearsI downgraded my firebase from 6.0.3 to 5.0.3 and error disappeared but when I firebase.auth().onAuthStateChanged((authenticate)=>{ if(authenticate){ this.props.navigation.replace("homeScreen") }else{ this.props.navigation.replace("signInScreen") } }) it shows Can't find variable :firebas
-
Gen1-1 over 4 yearsWith firebase functions, where can you specify the node --experimental-modules flag? I understand how to use the flag if running node from a command-line, but how can you specify it if you're using the firebase "serve" command?
-
damienix over 4 yearsAre you using TypeScript? In TS these modules work by default I think, maybe with a change in tsconfig. I haven't used firebase in JS (not TS) on Node.js actually...
-
MikhailRatner over 2 yearsSpent 2 damn hours searching to eventually find out that I missed the
/app';
in the first import statement. Thank you! Btw: can you explain why we need the "compat" addition? -
Kesava Karri over 2 yearsYeah I've spent sometime to come to a conclusion as well. The compat is for version 9 of firebase to be compatible with version 8. I guess they're going to change it from compat to modular for the next version. Reference link: firebase.google.com/docs/web/…