Where is pow function defined and implemented in C?
Solution 1
Quite often, an include file such as <math.h>
will include other header files that actually declare the functions you would expect to see in <math.h>
. The idea is that the program gets what it expects when it includes <math.h>
, even if the actual function definitions are in some other header file.
Finding the implementation of a standard library function such as pow()
is quite another matter. You will have to dig up the source code to your C standard runtime library and find the implementation in there.
Solution 2
Where it's defined depends on your environment. The code is inside a compiled C standard library somewhere.
Its "definition" is in the source code for your c standard library distribution. One such distribution is eglibc. This is browsable online, or in a source distribution:
Short answer: In the C standard library source code.
Solution 3
The actual implementation of pow
may vary from compiler to compiler. Generally, math.h (or a vendor-specific file included by math.h) provides the prototype for pow
(i.e., its declaration), but the implementation is buried in some library file such as libm.a. Depending on your compiler, the actual source code for pow
or any other library function may not be available.
Solution 4
declared: in the include directory of your system/SDK (e.g.: /usr/include;/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS3.2.sdk/usr/include/architecture/arm/math.h)
defined (implemented):
- as library (compiled, binary code): in the library directory of your system/SDK (e.g.: /usr/lib (in case of the math library it's libm.dylib)
- as source (program code): this is the interesting part. I work on a Mac OS X 10.6.x right now. The sources for the functions declared in math.h (e.g.: extern double pow ( double, double ); ) are not shipped with the installation (at least I couldn't find it). You are likely to find those sources in your system/SDK's C library. In my case the math library (libm) is a separate project, some of its sources are provided by Apple: http://www.opensource.apple.com/tarballs/Libm/Libm-315.tar.gz
The extern keyword in the function declaration of pow means, that it's defined somewhere else. Math functions are low-level high-performance implementations mostly done in assembly code (*.s). The assembly routines (taking the arguments/giving the parameters via registers/stack) are linked with the rest of the C library. The linking/exporting of the function/routine names is platform specific and doesn't really matter if ones goal is not dive into assembly coding.
I hope this helped, Raphael
Solution 5
If you are seeking how the calculation is implemented, you can find it here: http://fossies.org/dox/gcc-4.5.3/e__pow_8c_source.html The name of the function is __ieee754_pow which is called by pow function.
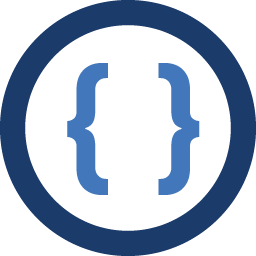
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I read that the pow(double, double) function is defined in "math.h" but I can't find its declaration.
Does anybody know where this function declared? And where is it implemented in C?
Reference:
http://publications.gbdirect.co.uk/c_book/chapter9/maths_functions.html
-
Admin over 14 yearsYes, I can use it but can't find its declaration. Can somebody find its declaration and implementation in math.h? Please post it here. thanks.
-
Admin over 14 yearsFor example, isless function is declared as: # ifndef isless # define isless(x, y) \ (extension \ ({ __typeof__(x) __x = (x); __typeof__(y) __y = (y); \ !isunordered (__x, __y) && __x < __y; })) # endif I expect similar thing that happens to pow(double, double)
-
Michael Burr over 14 yearsNote that most compilers do provide source to the library. But it can sometimes be quite a maze.
-
Chris Dodd over 4 yearsw_pow.c is not a very useful link as it basically just calls
__ieee754_pow(x, y);
which is the real definition...