Where is the Queue class in the Java Collections?
Solution 1
The Javadocs give a list of classes which implement Queue
.
All Known Implementing Classes:
AbstractQueue, ArrayBlockingQueue, ArrayDeque, ConcurrentLinkedQueue, DelayQueue, LinkedBlockingQueue, LinkedBlockingDeque, LinkedList, PriorityBlockingQueue, PriorityQueue, SynchronousQueue
There are also some subinterfaces which you might find useful:
All Known Subinterfaces:
BlockingDeque<E>, BlockingQueue<E>, Deque<E>
Solution 2
Queue has multiple implementations: from the API:
All Known Implementing Classes:
AbstractQueue, ArrayBlockingQueue, ArrayDeque, ConcurrentLinkedQueue,
DelayQueue, LinkedBlockingDeque, LinkedBlockingQueue, LinkedList,
PriorityBlockingQueue, PriorityQueue, SynchronousQueue
Note that AbstractQueue isn't a concrete class.
Some of these are from the package concurrent, so if you're implementing a jobqueue or something similar, you should go for ConcurrentLinkedQueue or PriorityBlockingQueue (for an heap) for ex.
Solution 3
The documentation for Queue
lists various implementations, including
Pick an implementation that suits your needs.
Solution 4
Queue<Integer> queue = new ArrayDeque<>();
queue.add(1);
queue.add(2);
queue.add(3);
while (!queue.isEmpty()) {
System.out.println(queue.remove());// prints 1 2 3
}
You can also use a LinkedList. But generally, for a Queue, ArrayDeque is preferred over LinkedList. Because ArrayDeque consumes lesser memory, is faster and doesn't allow nulls. Not allowing null is good, because if you do allow nulls, then when you do a peek() or poll() you could get a null even if the queue is not empty.
Solution 5
As well as using the API docs to find "all known implementing classes", there are often other non-public implementation that are nevertheless available through the public API (only without requiring reams of pointless documentation). If you click on "use" you will also find Collections.asLifoQueue (Deque
is already a Queue
, but it is FIFO rather than a stack).
Related videos on Youtube
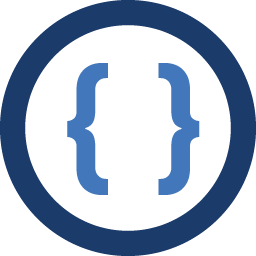
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I only see a Queue interface, is there no Queue class in the Java Collections?
-
James McMahon about 15 yearsAccording to java.sun.com/j2se/1.5.0/docs/api/java/util/ArrayList.html, ArrayList does not implement Queue.
-
arkap about 15 yearsWonder why people dont go to JavaDocs :P +1
-
Rob about 15 yearsYes, I realised that one when I went back to get a few links; obviously my memory is faulty.
-
James McMahon about 15 yearsIt happens. I'd be impressed if you have the entire Java library memorized. If you had EE memorized I'd back away slowly.
-
Rob about 15 yearsWell, there's javax.activation, javax.annotation, javax.ejb, and...wait, where are you going? There's so much more! ;)
-
palantus about 15 yearsEvery so often, I rediscover the "Use" pages and think, "Hey, this is great!" And then I forget them again.
-
Powerlord about 15 yearsFor the really lazy people, I'm going to note that all the ones with DeQue are double-ended queues.
-
Brett about 15 yearsThe "use" pages don't have the greatest of UIs.
-
Pieter over 13 yearsI'm confused. I just want a FIFO data structure that lets me enqueue and dequeue items as shown on Wikipedia and none of the specialized stuff. Which one do I pick? Isn't there a Java equivalent to C++'s STL queue?
-
palantus over 13 years@Pieter: Any of these classes will do the basic enqueue/dequeue operations. If you like, you can declare your queue as a Queue like
Queue<MyObject> myQueue = new LinkedList<MyObject>()
, which will force you to stick to the queue operations declared in the interface (like add/remove).