While loop to check character input
Solution 1
There is an error in your logic: you should be connecting those tests with AND: &&
:
while (choice != 'H' && choice != 'h' && choice != 'L' && ...
Furthermore choice
is never being updated within the body of the loop, so it will never change. Duplicate the choice = choiceString.charAt(0);
after you re-read choiceString
within the loop.
Conceptually, you want to keep asking for input so long as the character isn't H AND isn't L AND isn't S.
Solution 2
Use &&
instead of ||
.
while (choice != 'H' && choice != 'h' && choice != 'L' && choice != 'l' && choice != 'S' && choice != 's')
This can be simplified by converting to lower case first.
choice = Character.toLowerCase(choice);
while (choice != 'h' && choice != 'l' && choice != 's')
Solution 3
You never update choice
in your while
loop:
Just add this line at the end of your loop:
choice = choiceString.charAt(0);
Moreover you should check with &&
rather than ||
.
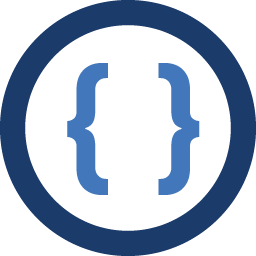
Admin
Updated on October 16, 2020Comments
-
Admin over 3 years
I am trying to accept input from the user in the form of a character. I have that working but I need to check and make sure it is one of 6 characters (H, h, S, s, L, l). I have a while loop but as soon as add more than one character statement to it, the loop gives the error for every value that should be correct.
Here is the function:
private static char getHighLow(Scanner keyboard) { System.out.println("High, Low, or Sevens (H/L/S): "); String choiceString = keyboard.next(); char choice = choiceString.charAt(0); while (choice != 'H' || choice != 'h' || choice != 'L' || choice != 'l' || choice != 'S' || choice != 's') { System.out.println("You have entered an invalid entry."); System.out.println("High, Low, or Sevens (H/L/S): "); choiceString = keyboard.next(); } return choice; }
What is the best way to continue checking for multiple characters like this?
-
Admin over 11 yearsPerfect that did it. I think I'm getting the logic for this now, but correct me if I'm wrong. I don't want to enter that loop unless none of those characters are entered. So it checks each character and fails because every single AND needs to be true?
-
Admin over 11 yearsPerfect. Thank you for the help!