Why constructor is used instead of functions?
Solution 1
The most important difference: When you instantiate an object it's constructor will be invoked whereas calling a method is always optional. You therefore might forget to call your initialization method and fail to initialize everything correctly.
For example, all these normal ways of instantiating an object will call the constructor
Foo* p = new Foo();
Foo p;
Or if you have mandatory arguments, don't define a default constructor and instead require construction with parameters:
class Foo
{
private:
Foo();
public:
Foo(int param1, double param2)
};
This has the advantage of requiring the arguments before you even instantiate the class. So you're forced to do:
Foo* p = new Foo(1, 5.0);
and failing to construct with valid arguments becomes a compiler error:
Foo* p = new Foo(); // compiler error
So whenever possible, always err on the side of doing your initialization in a constructor. There are a few cases where a constructor might not be viable. For example, the only way to fail a constructor is by using an exception. The failure to construct may be "routine" and not truly exceptional. Also exceptions might be expensive on some architectures. Another case might be when you want to ensure that virtual methods are fully bound, which is guaranteed to be true only after construction.
Solution 2
They can't both be used to initialize member variables. It is the job of the constructor to initialize members, and it is called automatically whenever you create a new instance.
Consider the following:
class Foo {
public:
// Constructor
Foo() : x(53) // Initialise x
{}
void bar() {
x = 42; // Error, attempt to *assign* a const member!
}
private:
const int x;
};
Without the constructor, there would be no way to initialise member x
.
Solution 3
Constructors are called automatically, so there's no need to worry whether the user has invoked an initialization method yet. However, the Google style guide does have something to say about constructors:
- There is no easy way for constructors to signal errors, short of using exceptions.
- If the work fails, we now have an object whose initialization code failed, so it may be an indeterminate state.
- If the work calls virtual functions, these calls will not get dispatched to the subclass implementations. Future modification to your class can quietly introduce this problem even if your class is not currently subclassed, causing much confusion.
- If someone creates a global variable of this type, the constructor code will be called before main(), possibly breaking some implicit assumptions in the constructor code.
Google's recommendation is to have straightforward initiation in a constructor, and non-trivial initiation in a separate method.
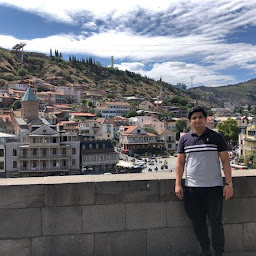
Rafay Zia Mir
Primarily a computational Physicist, secondarily a programmer.Love to work in both.Striving to learn learn and learn.I may be of little help but you can reach me at email: rafay_07[at]yahoo[dot]com
Updated on July 15, 2022Comments
-
Rafay Zia Mir almost 2 years
This is a very basic question, I searched it but i just want to ask this community that we have both
constructors
andmethods
. But normally we use constructor to initialize the variables instead of the methods. I think that both can be used to initialize variables. So what is the basic difference between both. Is there any solid reason? this is a very basic question so bear it for sake of beginner level. thanks in advance.. -
Oliver Charlesworth about 12 yearsWhich is all well and good, unless you're a fan of immutable objects.
-
Rafay Zia Mir about 12 yearsActually i am asking the initialization with some values demanded by user, let's suppose that for simulation purpose we have to initialize about 20 variables to the given values. If i initialize each variable correctly then in this case will you stick to your argument?
-
Doug T. about 12 years@kashmirilegion see my edits. If you need to initialize 20 variables you can either define a constructor that takes 20 variables or one that takes a struct.
-
chrisaycock about 12 yearsWhich is all well and good until you need to return an error code at runtime. :P
-
Rafay Zia Mir about 12 years+1 for your good answer. thank you your argument gave strength to mind to make a clear way about constructors.
-
vinod about 12 yearsThe main argument for following Google's C++ style guide seems to be the Big Name. I totally dislike their view on (not) using exceptions. If you don't use exceptions, you're using a very C-like style of C++. A well known C++ expert once said: www2.research.att.com/~bs/3rd_safe0.html To answer your bulleted list: (1) then just use exceptions (you just found out that there essential for using constructors/RAII) (2) again, you won't have an invalid object if you throw an exception (3) I agree, some nasty problems can arise, the compiler should warn you. (4) so, no code in constructors?