Why declaration by 'extern' doesn't work with static functions in C?
Solution 1
Why declaration by
extern
doesn't work withstatic
functions?
Because extern
indicates external linkage while static
indicates internal linkage. Obviously, You cannot have both on same function.
In simple words, when you use static
on the function you tell the compiler please limit the scope of this function only to this translation unit and do not allow anyone to access this from another translation unit.
while function declarations are extern
by default, when you specify extern
explicitly you tell the compiler, Please allow everyone from other translation units to access this function.
Well obviously the compiler cannot do both.
So make a choice, whether you want the function to be visible only in the translation unit or not.
If former make it static
and forget extern
. If latter just drop the static
.
Good Read:
What is external linkage and internal linkage?
Though above Q is for C++, most of what is discussed applies to C as well.
Solution 2
You declare it with static
static int foo(void);
static int foo(void)
{
return 0;
}
Solution 3
extern
means the function is defined in a different translation unit (file). static
means it is only used in the translation unit in which it is defined. The two are mutually exclusive.
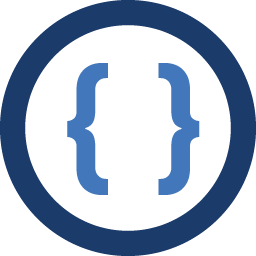
Admin
Updated on June 13, 2022Comments
-
Admin about 2 years
Suppose the code:
extern int foo(void); static int foo(void) { return 0; }
Try to compile with GCC
$ gcc -Wall -std=c99 1.c 1.c:3:12: error: static declaration of ‘foo’ follows non-static declaration 1.c:1:12: note: previous declaration of ‘foo’ was here 1.c:3:12: warning: ‘foo’ defined but not used [-Wunused-function]
So, how can I declare static function?