Why does the compiler complain with illegal start of expression error message?
Solution 1
These are called constants in Java and they should be defined in the class itself, not in its main method.
public static final int STARTINGYEAR = 1890;
.....
Now, if you remove the public static
, you can keep them in your main method but seems that's not what you intended. Also, this will make it impossible to use them in other methods as they will become local final variables of the main method.
Solution 2
There are several errors:
move static members out of the main method
DrawingPanel needs to be imported (in case its not in the same package)
Either change params of
nameToLowerCase(Scanner console, Scanner data)
to
nameToLowerCase(Scanner console)
or add the seconds parameter to its call
String nameFinal = nameToLowerCase(console);
To avoid such mistakes i recommend to use an ide like eclipse or intellij.
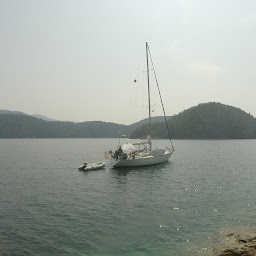
Marina Claire
Updated on June 04, 2022Comments
-
Marina Claire almost 2 years
This code looks through several files to find the popularity and meaning of whatever name you type in. The class constants that have errors are for a graph I have yet to make within the class. They are not being used right now, but will be soon.
I'm getting these errors:
BabyNames.java:8: error: illegal start of expression public static final int STARTINGYEAR = 1890; ^ BabyNames.java:8: error: illegal start of expression public static final int STARTINGYEAR = 1890; ^ BabyNames.java:8: error: ';' expected public static final int STARTINGYEAR = 1890; ^ BabyNames.java:9: error: illegal start of expression public static final int WIDTH = 60; ^ BabyNames.java:9: error: illegal start of expression public static final int WIDTH = 60; ^ BabyNames.java:9: error: ';' expected public static final int WIDTH = 60; ^ BabyNames.java:10: error: illegal start of expression public static final int HEIGHT = 30; ^ BabyNames.java:10: error: illegal start of expression public static final int HEIGHT = 30; ^ BabyNames.java:10: error: ';' expected public static final int HEIGHT = 30;
I think I have all the correct semi colons here, but I'm not sure what's going on Here is my code:
import java.util.*; import java.io.*; //import java.lang.*; public class BabyNames{ //ADD COMMENTS public static void main(String[] args) throws FileNotFoundException{ public static final int STARTINGYEAR = 1890; public static final int WIDTH = 60; public static final int HEIGHT = 30; Scanner console = new Scanner(System.in); DrawingPanel panel = new DrawingPanel(780,560); Graphics g = panel.getGraphics(); Scanner nameFile = new Scanner(new File("names.txt")); Scanner meaningsFile = new Scanner(new File("meanings.txt")); Scanner nameFile2 = new Scanner(new File("names2.txt")); intro(); //nameToLowerCase(console); if(STARTINGYEAR = 1890){ findingStatistics(console,nameFile); } else{ findingStatistics(console, nameFile2); drawGraph(g); } public static void intro(){ System.out.println("This program allows you to search through the"); System.out.println("data from the Social Security Administration"); System.out.println("to see how popular a particular name has been"); System.out.println("since 1890" ); System.out.println(); System.out.print("Name: "); } public static String nameToLowerCase(Scanner console, Scanner data){ String originalName = console.next(); String name = "" ; int lengthOfName = originalName.length(); String beginingOfName = originalName.substring(0,1).toUpperCase(); String endOfName = originalName.substring(1,lengthOfName).toLowerCase(); name = beginingOfName + endOfName; return name; } public static String findingStatistics(Scanner console, Scanner data){ String nameFinal = nameToLowerCase(console); boolean goesThroughOnce = false; // String statistics = ""; String currWord = ""; String currLine = ""; while (data.hasNext() && goesThroughOnce == false){ currLine = data.nextLine(); Scanner lineBeingRead = new Scanner(currLine); //make other scanners?? for each file currWord = lineBeingRead.next(); // if (currWord.equals(nameFinal) || currWord.equals(nameFinal.toUpperCase())){ // statistics = currLine; goesThroughOnce = true; System.out.println(statistics); } else{ } } return statistics; }