Why is === faster than == in PHP?
Solution 1
Because the equality operator ==
coerces, or converts, the data type temporarily to see if it’s equal to the other operand, whereas ===
(the identity operator) doesn’t need to do any converting whatsoever and thus less work is done, which makes it faster.
Solution 2
===
does not perform typecasting, so 0 == '0'
evaluates to true
, but 0 === '0'
- to false
.
Solution 3
There are two things to consider:
If operand types are different then
==
and===
produce different results. In that case the speed of the operators does not matter; what matters is which one produces the desired result.If operand types are same then you can use either
==
or===
as both will produce same results. In that case the speed of both operators is almost identical. This is because no type conversion is performed by either operators.
I compared the speed of:
$a == $b
vs$a === $b
- where
$a
and$b
were random integers [1, 100] - the two variables were generated and compared one million times
- the tests were run 10 times
And here are the results:
$a == $b $a === $b
--------- ---------
0.765770 0.762020
0.753041 0.825965
0.770631 0.783696
0.787824 0.781129
0.757506 0.796142
0.773537 0.796734
0.768171 0.767894
0.747850 0.777244
0.836462 0.826406
0.759361 0.773971
--------- ---------
0.772015 0.789120
You can see that the speed is almost identical.
Solution 4
First, === checks to see if the two arguments are the same type - so the number 1 and the string '1' fails on the type check before any comparisons are actually carried out. On the other hand, == doesn't check the type first and goes ahead and converts both arguments to the same type and then does the comparison.
Therefore, === is quicker at checking a fail condition
Solution 5
I don't really know if it's significantly faster, but === in most languages is a direct type comparison, while == will try to do type coercion if necessary/possible to gain a match.
Related videos on Youtube
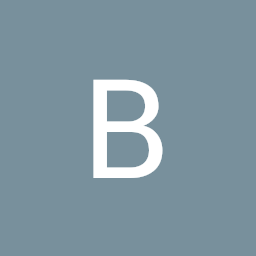
Comments
-
Bojan Muvrin over 3 years
Why is
===
faster than==
in PHP?-
Piskvor left the building about 14 yearsIt is faster, but is it significantly faster?
-
Kamil Szot about 14 yearsPlease don't read about what's faster in php. Read about how to get interesting data in single SQL query without abusing JOINs.
-
Marco Demaio over 13 yearsTo whom it might be interested in the same subject
=== vs ==
, but in JAVASCRIPT, can read here: stackoverflow.com/questions/359494/… -
Pacerier almost 11 years@Piskvor, that's not the question
-
Piskvor left the building about 10 years@Pacerier: Fair point - that's why I have only commented on this. It doesn't answer the question, but provides perspective on it.
-
pbarney over 3 yearsAbsolutely, we should avoid petty optimizations if they waste time that we could be using to learn proper SQL, but having this in your back pocket as a best practice is worth the few seconds it takes to comprehend the question. Most of us are programmers because we love to understand the inner-workings of things like this!
-
-
user1025901 about 14 yearsJavascript has the === operator.
-
pupeno about 14 yearsI'm sure you can do === in common lisp and scheme.
-
TomTom about 14 yearsJavascript - not in 3 langauge definitions I checked ;) And Lisp and Scheme are many things, but hardly common ;)
-
KitsuneYMG about 14 yearsruby has ===. It has been too long for me to remember if it does the same thing.
-
Chris about 14 yearsAlso, livedocs.adobe.com/flash/9.0/ActionScriptLangRefV3/… for actionscript. Basically, google "strict equality".
-
Bakhtiyor almost 14 yearsI think your opinion is contrary with the what PHP Manual says. They say $a == $b is TRUE if $a is equal to $b, where $a === $b is TRUE if $a is equal to $b, and they are of the same type.
-
Gromski almost 14 yearsI'd guess that
==
also checks the type first to see if any type conversion needs to be done. The fact that===
doesn't do any conversion in the following step is what makes it faster. -
Basic over 13 yearsI believe it's actually that the 2 operands point to the same area of memory for complex types but meder's answer encompasses that
-
Marco Demaio over 13 yearsIt makes sense (as it is in JS), but it would be nice if someone adds also a reference to some real simple performance tests.
-
Gung Foo over 11 yearsi wonder what happens if you do some billion iterations on a machine that isn't doing anything else and just output the average. looks like there is pretty much noise in here. ;)
-
awhie29urh2 about 11 yearsphpbench.com has an indication of performance difference between == and === under the "Control Structures" section.
-
Paul Spiegel over 6 yearsI came to the same conclusion: No difference could be messured if the operands are known to be from the same type. Other scenarios don't make sence. Almost all other answers are just wrong.
-
Pedro Amaral Couto about 6 yearsI believe this should have been the selected answer. It doesn't merely rationalise with assumptions, the assumptions were more ore less tested empirically.
-
Marco almost 5 years@PedroAmaralCouto I don't think so, since 10 is not an empirical study. The main reason there is near no difference is that the PHP compiler will probably optimize the code. One should use === unless type conversion is needed, it will help to reduce semantic error (even if it's once in your entire life). It also helps the next person reading the code what rules are enforced. You write once, it's read a few hundred times, if it can help clear up one person's doubt, it's already succeeded. Also no memory test if Empirical, since clone to same type. There are more resources than only time.
-
Pedro Amaral Couto over 4 years@Marco, when I say "empirical study", I mean it's based on experience, eg: running code instead of making an argument using only reason (or what's in your mind), without an experiment to back it up. Salman A values suggest === is equally sometimes a bit faster and sometimes a bit slower. This means the "Why is === faster than == in PHP?" begs the question: "How do you know === is faster than =="? Compiler optimizations is an explanation, not what is faster or slower and I didn't say what should be used.
-
Marco over 4 years@PedroAmaralCouto the answer given is 10 examples, which doesn't rule out a lot of external factors nor does Salman show how the examples were produced. I'm still pretty sure that the compiler would optimize them all not to have type conversion if possible, e.g. a quick type comparison is done first with type in memory, if you upped it to way larger numbers (of comparisons not number itself) where previous result can't be stored in the CPU cache, what result would you get? Or if you used e.g. BigInt/objects, etc. where custom variable types might "trick" the compiler.
-
SikoSoft over 4 yearsDo you only have "one" IF statement in your code base? That's weird because in every code base I've worked on, we have thousands of IF or comparative statements called everywhere.
-
Muhammad Omer Aslam over 4 yearsok then would there be any difference using just
if(value)
rather thanif(value===true)
orif(value==true)
? -
JPR over 4 years@MuhammadOmerAslam in
if(value)
thevalue
is directly converted to Boolean and then evaluated, while the strict comparison (===
) instead performs a comparison to obtain a Boolean which is then evaluated. The comparison probably takes longer in most if not all cases. But any performance benefit there should be insignificant and our time better spent either on solving problems more efficiently or on just making the code more readable. -
8ctopus over 3 yearsI actually found a significant difference: === being 15% faster than ==. see here stackoverflow.com/a/65126758/10126479
-
Salman A over 3 years@8ctopus are you comparing two identical datatypes in those tests?
-
8ctopus over 3 years@SalmanA no, the comparison is between a bool and an int so conversion needs to occur.