Why show java.lang.ClassCastException: android.text.SpannableString cannot be cast to java.lang.String?
Solution 1
SpannableString is not String directly. so, you can not cast. but, it can be converted to string. you can convert something to string with concatenating with empty string.
pasteData = "" + item.getText();
Solution 2
Returns a string with the same characters in the same order as in this sequence.
You need to use next code.
String pasteData = item.getText().toString();
You can not cast to android.text.SpannableString
because item.getText()
returns CharSequence
, there are a lot of implementations of it
Solution 3
This is what it worked for me in Kotlin
:
val str = text.toString()
Solution 4
If your Spanned text only containing HTML content then you can convert it using Html.toHtml()
String htmlString = Html.toHtml(spannedText);
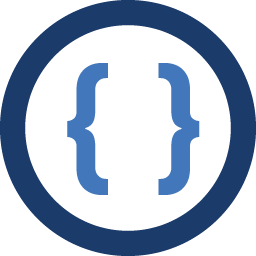
Admin
Updated on July 23, 2022Comments
-
Admin almost 2 years
When copying
String
from any browser page,pasteData
works properly. However when copyingSpannedString
from a message sent item editor(field), the application crashes and shows this error message:java.lang.ClassCastException: android.text.SpannableString cannot be cast to java.lang.String
My code:
// since the clipboard contains plain text. ClipData.Item item = clipBoard.getPrimaryClip().getItemAt(0); // Gets the clipboard as text. String pasteData = new String(); pasteData = (String) item.getText();
where the
ClipboardManager
instance defined asclipBoard
, below:clipBoard = (ClipboardManager) context.getSystemService(context.CLIPBOARD_SERVICE); clipBoard.addPrimaryClipChangedListener(new ClipboardListener());
All I'm trying to do is use
pasteData
inString
format. How to get rid of this error? Any help is appreciated.