window.location Does not work in javascript
Solution 1
change js to:
function checkAnswers()
{
var myQuiz=document.getElementById("myQuiz");
if (myQuiz.elements[1].checked) {
window.location.href = "http://www.google.com";
}
else {
alert("Failed");
}
return false;
};
and change:
<form id="myQuiz" onsubmit="checkAnswers()" action="">
to
<form id="myQuiz" onsubmit="return checkAnswers();" action="">
Solution 2
When you change the location, you must give it an absolute URL:
location.href = '/some_page_on_my_site';
Or:
location.href = 'http://www.google.com';
Or:
location.href = '//www.google.com';
The last one will go to http or https, depending the current scheme. Thanks @Derek
Solution 3
2018
multibrowser: safari, chrome, firefox:
just this work:
window.location.replace(url)
another options that tries assign url to window.location or window.location fails
Solution 4
When you run this:
window.location ="www.google.com";
You're running this relative to the current requestURI. Thus, if you are on the http://example.com page, then you're attempting to redirect to:
http://example.com/www.google.com
Instead, just remember to include the protocol:
window.location ="http://www.google.com";
Remember, window.location is not your address bar, designed to deal with nontechnical people entering a web address in an address bar. With window.location, you must explicitly include http://.
Solution 5
Your url used in window.location
is local; you need to add in the http://
part of the url.
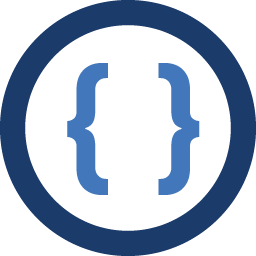
Admin
Updated on July 06, 2020Comments
-
Admin almost 4 years
This code below for some reason doesn't work when trying to redirect. I have tried several other ways such as window.location.href and more. The only thing that works is
window.open ("")
but this opens a page in a new window when I need it to be on the same. If I dowindow.open("", "_self")
then it does not work again. If I replace thewindow.location
with analert
it works fine, so I think the overall code is normal just something preventing it from redirecting on the same page. This issue is also on my Windows Chrome and a Mac Firefox.<html> <head> <script type="text/javascript"> function checkAnswers(){ getElementById("myQuiz"); if(myQuiz.elements[1].checked){ window.location = "www.google.com"; }else{ alert("Failed"); } }; </script> </head> <body> <img src="Castle.JPG" /> <form id="myQuiz" onsubmit="checkAnswers()" action=""> <p>Name the country this castle is located in? <br/> <input type="radio" name="radiobutton" value="A"/> <label>England</label> <input type="radio" name="radiobutton" value="B"/> <label>Ireland</label> <input type="radio" name="radiobutton" value="C"/> <label>Spain</label> <input type="radio" name="radiobutton" value="D"/> <label>France</label> <input type="submit" name="submit" value="Submit"/> <input type="reset" name="reset" value="Reset"/> </p> </form> </body> </html>
-
Admin almost 12 yearsThanks! Adding the return is definitely what fixed it. I'm obviously very new to javascript, (like a few days new), so can you tell me why this worked? Thanks again.
-
Derek 朕會功夫 almost 12 years@GeorgeKashouh - You have to return, the value of the function
checkAnswers
has returned, toonsubmit
. -
jamesmortensen almost 12 yearsTo expand, when you return false in an onsubmit, the form doesn't submit. In other words, the page only redirects if the element is checked.