Windows keystores and certificates
Maybe you want to take a look at Oracle's documentation [1] on the SunMSCAPI
provider, that can be used to access certificates and keys stored in the Windows-MY
(Personal) and Windows-ROOT
(Trusted Root Certification Authorities) stores.
There is a little code snippet as well, which seems to match your needs quite reasonably:
KeyStore ks = KeyStore.getInstance("Windows-MY");
// Note: When a security manager is installed,
// the following call requires SecurityPermission
// "authProvider.SunMSCAPI".
ks.load(null, null);
byte[] data = ...
String alias = "myRSA";
PrivateKey privKey = (PrivateKey) ks.getKey(alias, null);
Certificate cert = ks.getCertificate(alias);
Provider p = ks.getProvider();
Signature sig = Signature.getInstance("SHA1withRSA", p);
sig.initSign(privKey);
sig.update(data);
byte[] signature = sig.sign();
System.out.println("\tGenerated signature...");
sig.initVerify(cert);
sig.update(data);
if (sig.verify(signature)) {
System.out.println("\tSignature verified!");
}
Summarizing: The Windows-My store holds certificates as well as private keys, both can be read from Java using the SunMSCAPI
provider and can be used to sign digital documents.
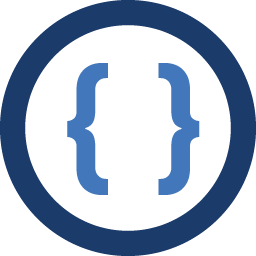
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I've recently inherited a project with minimal documentation that performs digital signatures of documents and I've received a change request that has left me a little baffled.
The application is Java based, and makes use of Java Keystores (JKS) and uses the private key of the alias specified as a command line operation to digitally sign an input document. This all appears fairly straightforward to me, however the change request has left me confused.
The client has requested the ability to use "Windows" keystores (more specifically, the Windows-MY keystore which relates to personal certificates as far as I can tell). Now, my initial assumption is that what the client is requesting is simply not possible as this key store will only ever contain certificates, which can not be used for signing documents in any capacity. Am I incorrect, or will the Windows-MY keystore only ever contain a public certificate? I don't believe a private key would ever be embedded within one of these certificates.
Unfortunately there are a some communication difficulties so I'd like to make sure my reasoning is correct before proceeding any further.
Here's some more evidence to support my case (communication from the client):
Creating Windows Key Store (Exporting from Java Keystore ) steps are here -
generate RSA key
keytool -genkey -alias mykey -keyalg RSA -keystore my.jks -keysize 2048
Export Certificate from the above keystore:
keytool -export -alias mykey -file mykey.crt -keystore my.jks Enter keystore password: temp123 Certificate stored in file <mykey.crt>
Install the above certificate in windows keystore.
a. Double click on “mykey.crt” and click on Install certificate
b. Select “Place all certificates in the following store” radio button and click “Browse” button to Added it in windows Certificate store. c. Check this certificate in WindowsMy store.
Unless I'm wrong, keytool will only ever generate a certificate type rather than an actual public/private key pair?
Any assistance or even affirmation would be greatly appreciated, apologies for the lack of clarity in the question but unfortunately this is all I have to work with at the moment. Java or Windows specific answers would be helpful but even just confirmation of the basic principles would be appreciated.
Thanks in advance