With flutter printing package, how do I print a PDF from a URL?
I have tried the following ways to achieve the printing feature:
-
Directly get the remote data bytes
With the popular package printing
var data = await http.get(url);
await Printing.layoutPdf(onLayout: (_) => data.bodyBytes);
But I found that this way will have the printing area disorder issue when printing a roller size paper (POS printer)
-
Sharing (alternative of the first one)
Use the sharePdf function
var data = await http.get(url);
await Printing.sharePdf(bytes: data.bodyBytes, filename: 'my-document.pdf');
This one needs an additional step to print (click the 'functions' icon of browser then print)
-
View it from the browser and print it natively
Check out this package url_launcher
import 'package:url_launcher/url_launcher.dart';
if (await canLaunch(url)) {
await launch(url);
}
This one needs an additional step to print (click the 'functions' icon of browser then print)
-
Download the remote file and save it, use the flutter_pdf_printer package.
Use this package flutter_pdf_printer and path_provider
var data = await http.get(url);
final output = await getTemporaryDirectory();
final file = File('${output.path}/your_file_name_${new DateTime.now().microsecondsSinceEpoch}.pdf');
await file.writeAsBytes(data.bodyBytes);
await FlutterPdfPrinter.printFile(file.path);
*** this package will have channel registrant issue, please run flutter clean
after installation ***
This package is a little bit outdated, but it works for me to print with a roller 80 size pdf. It uses the Swift UIKit package, which has good compatibility.
Summary
My scenario is to print a roller 80 size pdf with infinite height, the printing package doesn't work for cuz it cannot preview the pdf properly. So I changed to the last way to print, which works for me. If you just print an A4 or a regular size PDF, the printing package is still a good choice.
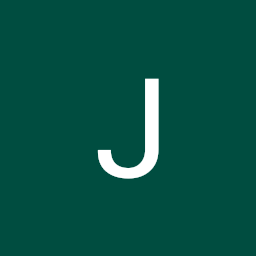
user11015833
Updated on December 16, 2022Comments
-
user11015833 over 1 year
I am using the printing package in flutter: https://pub.dev/packages/printing
The documentation shows how to create PDFs from scratch. I already have a PDF at a URL. Is there a way to retrieve it and print it with the package? Or is there an alternate method with another package?