Word Automation: Replace an Image using C#
19,082
Solution 1
You can loop through the InlineShapes and replace the pictures
using System.Collections.Generic;
using Word = Microsoft.Office.Interop.Word;
namespace WordExample
{
class WordExample
{
#region Constructor
public WordExample()
{
WordApp = new Microsoft.Office.Interop.Word.Application();
}
#endregion
#region Fields
private Word.Application WordApp;
private object missing = System.Reflection.Missing.Value;
private object yes = true;
private object no = false;
private Word.Document d;
private object filename = @"C:\FullPathToFile\example.doc";
#endregion
#region Methods
public void UpdateDoc()
{
d = WordApp.Documents.Open(ref filename, ref missing, ref no, ref missing,
ref missing, ref missing, ref missing, ref missing, ref missing,
ref missing, ref missing, ref yes, ref missing, ref missing, ref missing, ref missing);
List<Word.Range> ranges = new List<Microsoft.Office.Interop.Word.Range>();
foreach (Word.InlineShape s in d.InlineShapes)
{
if (s.Type == Microsoft.Office.Interop.Word.WdInlineShapeType.wdInlineShapePicture)
{
ranges.Add(s.Range);
s.Delete();
}
}
foreach (Word.Range r in ranges)
{
r.InlineShapes.AddPicture(@"c:\PathToNewImage\Image.jpg", ref missing, ref missing, ref missing);
}
WordApp.Quit(ref yes, ref missing, ref missing);
}
#endregion
}
}
Solution 2
Do you want to replace a shape or inline shape ? This is a big difference!
For an inline shape there are plenty of examples on the net. For a shape you can do like this :
private object missing = System.Reflection.Missing.Value;
.....other code.....
foreach (Microsoft.Office.Interop.Word.Shape s in wordApp.ActiveDocument.Shapes)
{
if (s.AlternativeText.ToUpper().Contains("FOTO"))
{
object A = s.Anchor;
Shape new = Brief.Shapes.AddPicture(@"mynewpicture.jpg", ref missing, ref missing, ref missing, ref missing, ref missing, ref missing,ref A);
new.Top = s.Top;
new.Left = s.Left;
new.Width = s.Width;
new.Height = s.Height;
s.Delete();
}
}
Solution 3
I just copy some path from @Mario Favere and make more easy
- First create Blank Picture and Insert Picture into word Document
- Second need to add Alt Text right click on Picture
private object missing = System.Reflection.Missing.Value;
.....other code.....
// Change Image
foreach (Microsoft.Office.Interop.Word.Shape s in wordApp.ActiveDocument.Shapes)
{
if (s.AlternativeText.ToUpper().Contains("POTO"))
{
s.Fill.UserPicture(@"PATH");
}
}
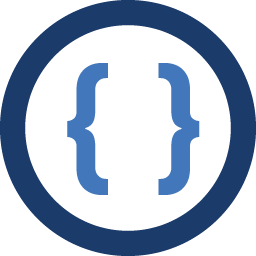
Author by
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
I'm trying to change text and images in a word document using c# and word automation. I've got it working fine for text where I do something like the snippet below, but I don't even know how to start for replacing the image.
Any help is greatly appreciated!
Oliver
using Microsoft.Office.Interop.Word; ... private static Application WordApp; private static object missing = System.Reflection.Missing.Value; private static object yes = true; private static object no = false; ... object search; object replace; object replaceAll = Microsoft.Office.Interop.Word.WdReplace.wdReplaceAll; object filename = SourceFile; object destination = DestinationFile; Document d = WordApp.Documents.Open( ref filename, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing, ref missing); d.Activate(); search = "OLDSTRING"; replace = "NEWSTRING"; WordApp.Selection.Find.Execute( ref search, ref yes, ref yes, ref no, ref no, ref no, ref yes, ref missing, ref missing, ref replace, ref replaceAll, ref missing, ref yes, ref missing, ref missing);
-
Ariel Gimenez almost 13 yearsExcelent code! But if its possible to identificate a picture into the loop? this is because i need to replace only one image into my automation project thanks in advance
-
Chris about 8 yearsI know this is really old, but the only way you can really tell shapes apart is by adding custom info to it. You can add a hyperlink and use the address, or use the
Title
orAlternativeText
properties (these are visible in theFormat Picture
dialog, underAlt Text
).