Working with the Google Location API
Solution 1
Fully documented .NET library -
Google Maps Web Services API wrapper for .NET https://github.com/maximn/google-maps/
//Directions
DirectionsRequest directionsRequest = new DirectionsRequest()
{
Origin = "NYC, 5th and 39",
Destination = "Philladephia, Chesnut and Wallnut",
};
DirectionsResponse directions = MapsAPI.GetDirections(directionsRequest);
//Geocode
GeocodingRequest geocodeRequest = new GeocodingRequest()
{
Address = "new york city",
};
GeocodingResponse geocode = MapsAPI.GetGeocode(geocodeRequest);
Console.WriteLine(geocode);
//Elevation
ElevationRequest elevationRequest = new ElevationRequest()
{
Locations = new Location[] { new Location(54, 78) },
};
ElevationResponse elevation = MapsAPI.GetElevation(elevationRequest);
Console.WriteLine(elevation);
Solution 2
Here is a sample code to get what you want
using System;
using System.Web;
using System.Net;
using System.Runtime.Serialization.Json;
namespace GoogleMapsSample
{
public class GoogleMaps
{
/// <summary>
///
/// </summary>
/// <param name="address"></param>
/// <returns></returns>
public static GeoResponse GetGeoCodedResults(string address)
{
string url = string.Format(
"http://maps.google.com/maps/api/geocode/json?address={0}®ion=dk&sensor=false",
HttpUtility.UrlEncode(address)
);
var request = (HttpWebRequest)HttpWebRequest.Create(url);
request.Headers.Add(HttpRequestHeader.AcceptEncoding, "gzip,deflate");
request.AutomaticDecompression = DecompressionMethods.GZip | DecompressionMethods.Deflate;
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(GeoResponse));
var res = (GeoResponse)serializer.ReadObject(request.GetResponse().GetResponseStream());
return res;
}
}
and you helper class would be
namespace GoogleMapsSample
{
[DataContract]
public class GeoResponse
{
[DataMember(Name = "status")]
public string Status { get; set; }
[DataMember(Name = "results")]
public CResult[] Results { get; set; }
[DataContract]
public class CResult
{
[DataMember(Name = "geometry")]
public CGeometry Geometry { get; set; }
[DataContract]
public class CGeometry
{
[DataMember(Name = "location")]
public CLocation Location { get; set; }
[DataContract]
public class CLocation
{
[DataMember(Name = "lat")]
public double Lat { get; set; }
[DataMember(Name = "lng")]
public double Lng { get; set; }
}
}
}
public GeoResponse()
{ }
}
}
Then you can use this code in your control/winforms like this
GeoResponse response = new GeoResponse();
response = GoogleMaps.GetGeoCodedResults(TextBoxPostcode.Text);
and build the JS on the code behind using StringBuilder or anyting else
Solution 3
The Geocode API is rather simple, to get lat/lon from the api you only need to 3 params: output, sensor and address.
output the output format you want, json or xml (IIRC)
sensor should be a boolean indicating weather or not the value comes from a sensor such as a GPS chip.
address should be the address (don't forget to url encode it) you wish to geocode.
This is an example, where I geocode my office address, and get JSON in response: http://maps.googleapis.com/maps/api/geocode/json?sensor=false&address=1+Maritime+Plaza+San+Francisco+CA
If you navigate to that you should see something like:
{
"status": "OK",
"results": [ {
"types": [ "street_address" ],
"formatted_address": "1 Maritime Plaza, San Francisco, CA 94111, USA",
"address_components": [ {
"long_name": "1",
"short_name": "1",
"types": [ "street_number" ]
}, {
"long_name": "Maritime Plaza",
"short_name": "Maritime Plaza",
"types": [ "route" ]
}, {
"long_name": "San Francisco",
"short_name": "San Francisco",
"types": [ "locality", "political" ]
}, {
"long_name": "San Francisco",
"short_name": "San Francisco",
"types": [ "administrative_area_level_3", "political" ]
}, {
"long_name": "San Francisco",
"short_name": "San Francisco",
"types": [ "administrative_area_level_2", "political" ]
}, {
"long_name": "California",
"short_name": "CA",
"types": [ "administrative_area_level_1", "political" ]
}, {
"long_name": "United States",
"short_name": "US",
"types": [ "country", "political" ]
}, {
"long_name": "94111",
"short_name": "94111",
"types": [ "postal_code" ]
} ],
"geometry": {
"location": {
"lat": 37.7953907,
"lng": -122.3991803
},
"location_type": "ROOFTOP",
"viewport": {
"southwest": {
"lat": 37.7922431,
"lng": -122.4023279
},
"northeast": {
"lat": 37.7985383,
"lng": -122.3960327
}
}
}
} ]
}
If you take the lat/lon provided and place it on a map you see a pointer on my office building.
Solution 4
I recently had to build a Store Locator for a project I am working on. I have never before this used any of the Google or Bing API's. Using this tutorial I was able to get a pretty good grasp of the Location API. I would suggest going through this tutorial and by the end of the three tutorials you should have a good understanding.
Building a Store Locator ASP.NET Application Using Google Maps API (Part 1)
Building a Store Locator ASP.NET Application Using Google Maps API (Part 2)
Building a Store Locator ASP.NET Application Using Google Maps API (Part 3)
Solution 5
By using GuigleAPI nuget you can simply do:
GoogleGeocodingAPI.GoogleAPIKey = "YOUR API KEY";
var result = await GoogleGeocodingAPI.GetCoordinatesFromAddressAsync("100 Market St, Southbank");
var latitude = result.Item1;
var longitude = result.Item2;
Just install it with the nuget command Install-Package Easyforce.GuigleAPI and you're good to go.
Check this answer for more details: https://stackoverflow.com/a/44484010/1550202
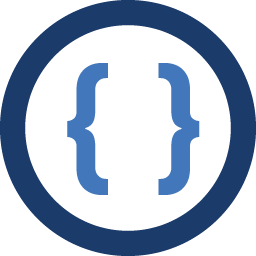
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Forgive me for my ignorance, but after several hours of searching, I'm having little luck.
Anyway, I am attempting to write a small desktop application that will allow a user to enter an address and then have their approximate location returned in GPS coordinates. From what I can tell, Google provides a geocoding API[1] that allows requests in the following form:
http://maps.googleapis.com/maps/api/geocode/output?parameters
While I'm familiar with writing basic C Sharp applications, I really have no idea where to start when it comes to interfacing with this API. Any help that you guys could provide would be greatly appreciated.
-
Admin over 13 yearsI'll be sure to try this out. I just wanted to say thanks in the meantime, I really appreciate it.
-
Cees Timmerman about 12 yearsIf you add the optional region parameter, it will try harder to return a location there.
-
czetsuya almost 12 yearsAnybody tried this API on MVC3 c# app? In my side it's loading page for a long time :-? But when I run a console app everything is working fine.
-
Killnine almost 12 years@czetsuya I had the same problem with the latest version on GitHub last night. just appears to hang and never recover...
-
Maxim almost 12 yearsWe just fixed it few days ago. Please make sure you're running on the latest version. In addition, I'll upload a nuget package in the next days.
-
Johann almost 12 years@Maxim - The library is awesomely easy to use, thanks :) I'm still having this issue though, has the NuGet package been updated with a version that fixes this problem? I'm not running with an Enterprise license yet, but we are planning on purchasing one when we get everything working.
-
Brendon Bezuidenhout about 11 yearsThanks for this @Maxim - One thing I'm curious about is how to change the unit system from metric to imperial for instance?
-
Maxim about 11 yearsThis is just wrapping Google's Maps API, it doesn't add any functionality. As I remember they don't support it but you can do it very easily in your code.