WPF Boolean Trigger
21,899
Solution 1
in *.cs file:
public partial class MainWindow : INotifyPropertyChanged
{
public MainWindow()
{
DataContext = this;
InitializeComponent();
}
public event PropertyChangedEventHandler PropertyChanged = delegate { };
public bool Flag { get; set; }
private void ButtonClick(object sender, RoutedEventArgs e)
{
Flag = true;
OnPropertyChanged("Flag");
}
protected void OnPropertyChanged(string property)
{
PropertyChanged(this, new PropertyChangedEventArgs(property));
}
}
in xaml form:
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow"
Width="525"
Height="350">
<Window.Resources>
<Style TargetType="Grid">
<Style.Triggers>
<DataTrigger Binding="{Binding Flag}" Value="True">
<Setter Property="Background" Value="Red" />
</DataTrigger>
</Style.Triggers>
</Style>
</Window.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="30" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Button Click="ButtonClick" Content="Click Me" />
</Grid>
</Window>
Solution 2
You can use a DataTrigger. I think you need to use it within a style or template though.
Alternatively, you can capture the changes in the code behind.
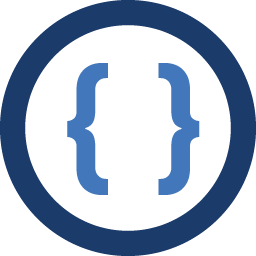
Author by
Admin
Updated on March 08, 2020Comments
-
Admin about 4 years
I have a boolean in the Window class of my WPF application. How can I target a trigger depending on whether this boolean is true or false?
<Grid> <Grid.Triggers> <Trigger ... /> </Grid.Triggers> </Grid>
Thanks.