WPF CommandParameter in Textbox
24,216
Solution 1
If i want the command to be executed if the user presses enter, i like to use this. Note the clever use of the IsDefault Binding :-)
<TextBox x:Name="inputBox"/>
<Button Command="{Binding CutCommand}"
CommandParameter="{Binding Text, ElementName=inputBox}"
Content="Cut"
IsDefault="{Binding IsFocused, ElementName=inputBox}" />
If you don't want the button to be visible, you can set its visibility to collapsed of course. I think it'll still execute the command if you hit enter.
Solution 2
This code works for me
<UserControl x:Class="Test"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
Height="Auto" Width="Auto">
<UserControl.InputBindings>
<KeyBinding Key="Enter" Command="{Binding ScanCommand}" CommandParameter="{Binding Text, ElementName=tbBarcode}"/>
</UserControl.InputBindings>
<Grid Name="LayoutRoot">
<TextBox x:Name="tbBarcode" Height="23"/>
</Grid>
</UserControl>
Solution 3
What have you tried? This code works for me:
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="300" Width="300">
<Window.CommandBindings>
<CommandBinding Command="Cut" Executed="CommandBinding_Executed" />
</Window.CommandBindings>
<StackPanel>
<TextBox x:Name="textBox1" />
<Button Command="Cut"
CommandParameter="{Binding Text,ElementName=textBox1}"
Content="Cut" />
</StackPanel>
</Window>
With this event handler:
private void CommandBinding_Executed(object sender, ExecutedRoutedEventArgs e)
{
MessageBox.Show(e.Parameter.ToString());
}
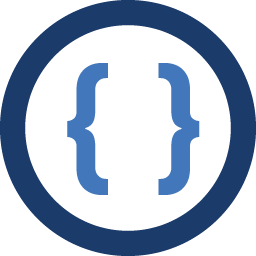
Author by
Admin
Updated on May 19, 2020Comments
-
Admin about 4 years
I am using MVVM pattern and i have a textbox in parent window and want to send some text to the popup window which will appear on Textchanged.
I tried using commandparameter but it is not working for me.
Please help..
Thanks Sharath
-
Admin about 15 yearsI want to use textbox textchanged or when user clicks enter on textbox from keyboard. Don't want to button.
-
Matt Hamilton about 15 yearsIf you're using a Command then you have to use an ICommandSource like a Button. Commands aren't the same as event handlers.
-
Admin about 15 yearsCan i do the same for listbox. I mean when i Double click on the listbox items. The button click even should be raised.
-
Botz3000 about 15 yearsI don't know. I invoked the command manually in code-behind for that. I'm pretty new to xaml though, so who knows.
-
Pavlin Marinov almost 5 yearsI think setting button's visibility to hidden (not collapse) will do the job.
-
nulltron over 4 years@Botz3000 you're the real mvp