write header rows to csv python
import csv
import os
file_name = 'C:\\fa.csv'
fake_data = [[1, 2, 3, 4, 5, 4444, 6, 67], [1, 2, 3, 4, 5, 4444, 6, 67],
[1, 2, 3, 4, 5, 4444, 6, 67], [1, 2, 3, 4, 5, 4444, 6, 67]]
headers = ['DataA', 'DataB', 'DataC', 'DataD']
if os.path.isfile(file_name):
with open(file_name, 'a', encoding="utf-8") as outfile:
writer = csv.writer(outfile)
for datum in fake_data:
writer.writerow(datum)
else:
with open(file_name, 'w', encoding="utf-8") as outfile:
writer = csv.writer(outfile)
writer.writerow(headers)
for datum in fake_data:
writer.writerow(datum)
In the first line open('C:\\fa.csv', 'a+', newline='', encoding="utf-8")
you have a+
which is append you want w
which is write.
Check this out: https://docs.python.org/3/tutorial/inputoutput.html#reading-and-writing-files
Related videos on Youtube
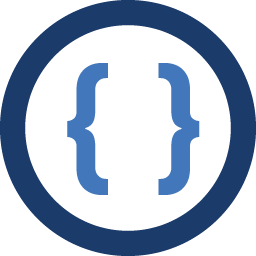
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
How do I add headers to row one in a csv?
My solution currently appends everything
Something like:
writer.writerow(['DataA', 'DataB', 'DataC', 'DATA D'])[0]
I feel like there an easy way of doing this and I'm overlooking the obvious.
I've looked at a lot of examples online but am still struggling as to how you can easily do this in a csv.
As an example lets say I wanted to scrape data of SO - load up selenium, write that into four columns and do this for 20 pages. I'd want to write the headers to row 1 each time and then append the scraped data
with open('C:\\fa.csv', 'a+', newline='', encoding="utf-8") as outfile: writer = csv.writer(outfile) writer.writerow(['DataA', 'DataB', 'DataC', 'DataD']) for row in zip(ZAW_text, RESULTS1, RESULTS): writer.writerow(row) #writer.writerows({'Date': row[0], 'temperature 1': row[1], 'temperature 2': 0.0} for row in writer) print(row)
-
AtHeartEngineer over 6 yearsThat is pretty much how you do it. I don't think there is an easier way.
-
Admin over 6 years@Tyler.Exposure Very well. Well in my case it appends the headers so it's not working for me. How do I get around that as I want headers in row one.
-
Admin over 6 yearsPretty sure I'm missing something. You can specify columns in csv surely rows is possible too.
-
AtHeartEngineer over 6 yearsAHHH I see what you did. I'm posting an answer.
-
-
Admin over 6 yearsI see. In the example with 20 other pages, will that data still be appended in the csv. Or will it all overwrite each other.
-
Admin over 6 yearsUnfortunately. The headers need to be at row 1. The data needs to be appended as its in a loop
-
AtHeartEngineer over 6 yearsEvery time you run this whole function, it will overwrite the file, but as its going through the for loop it will append, because you are writing a new row each time. Make sense?
-
Admin over 6 yearsUh.. that makes sense. I'd rather not overwrite the file if possible and keep an ever growing list of data. Is that possible? Hmm
-
AtHeartEngineer over 6 yearsSo, this basically checks if the file exists, if it exists, then it will only append to it, if it doesn't exist, it will write the header row, then continue writing new rows to it.
-
AtHeartEngineer over 6 yearsLet us continue this discussion in chat.
-
Loy about 5 yearsYou can also just write a line into outfile containing the header before instantiate csv.writter object.