Write Python OrderedDict to CSV
Solution 1
Alright, I'm going to answer my own question here. A couple of people were kind enough to offer suggestions in the comments. As suggested, I was working on accomplishing this with Pandas. As I was doing so, however, it occurred to me that I could do this without having to learn the ins and outs of the Pandas module. Here's what I came up with:
import csv
keys, values = [], []
for key, value in myOrderedDict.items():
keys.append(key)
values.append(value)
with open("frequencies.csv", "w") as outfile:
csvwriter = csv.writer(outfile)
csvwriter.writerow(keys)
csvwriter.writerow(values)
So here's what's going on here:
Create two empty lists corresponding to the keys and values in my ordered dictionary
Iterate over the key/value pairs in my ordered dictionary, appending each pair to its respective list. Because lists in Python retain their order, this ensures that items of corresponding indices in either list belong together
Write the keys to the first row of my CSV, and the values to the second
I'm sure there are more elegant ways to do this, but this is is sufficient for my purposes.
Solution 2
As of Python 3.7 dictionaries retain order so you can just use dict() to turn an ordered dictionary into a usable dictionary.
with open("frequencies.csv", "w") as outfile:
csvwriter = csv.writer(outfile)
csvwriter.writerow(dict(myDict))
csvwriter.writerow(dict(myDict).values())
Solution 3
Here is another, more general solution assuming you don't have a list of rows (maybe they don't fit in memory) or a copy of the headers (maybe the write_csv
function is generic):
def gen_rows():
yield OrderedDict(a=1, b=2)
def write_csv():
it = genrows()
first_row = it.next() # __next__ in py3
with open("frequencies.csv", "w") as outfile:
wr = csv.DictWriter(outfile, fieldnames=list(first_row))
wr.writeheader()
wr.writerow(first_row)
wr.writerows(it)
Related videos on Youtube
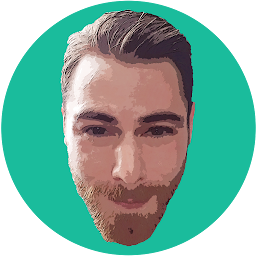
jguberman
I am an undergraduate Psychology student at the Illinois Institute of Technology (Illinois Tech). I am interested in developing assistive technologies for individuals with mental illnesses and other cognitive and/or physical disabilities. Currently, I am conducting a preliminary review of the literature regarding display design and head-up displays, and I am interested in designing augmented reality (AR) tools to assist individuals with autistic-spectrum disorders navigate the social world. My other research interests include: the use of peripheral devices to create AR experiences that increase mindfulness and overall psychological well-being, the use of artificial intelligences to mitigate the effects of dementia and/or executive functioning disorders, and the psychology of artificial intelligences. After earning my Bachelor’s degree from Illinois Tech, I plan to go on to earn advanced degrees in human-computer interaction.
Updated on June 04, 2022Comments
-
jguberman almost 2 years
I have an ordered dictionary that, as one would expect, contains a number of key-value pairs. I need to parse the contents of the ordered dictionary into CSV format, with the keys in the top row and their respective values in the second row. I've got the first row down, but I'm at a loss as to how to write the second row. The values in the OrderedDict are what you'd expect (what it looks like printed out in the terminal):
([(a, 1), (b, 2), (c, 3)])
. Here's what I have:import csv with open("frequencies.csv", "w") as outfile: csvwriter = csv.writer(outfile) csvwriter.writerow(myDict)
I tried iterating over the values and appending them to a list, but the list didn't maintain the original order of the ordered dictionary, which, as far as I could tell, would have made it pretty difficult to print the values from the list to the CSV so that they'd match up with the correct keys.
Thanks in advance for your help!
-
Marat over 7 yearsdid you try
csv.DictWriter
?
-
-
colidyre over 5 yearsOP does not want an unordered dict.
-
Frederick Chute over 5 yearsOP wants to "maintain the original order of the ordered dictionary" in his csv which this code does.
-
colidyre over 5 yearsHow is the ordering kept by using
dict()
-function? -
Frederick Chute over 5 yearsIts a language feature in python 3.7
-
colidyre over 5 yearsThank you for clarifying it! It would be nice to add the information about the version into answer's text. The question was asked on december 2016. I think, Python 3.5 was the latest version here without any inserted order on dicts which come into play on 3.6 (without reliability), I think (not 100% sure about this) and is considered as language feature by Guido on 3.7 - - that's right. :)
-
Nagabhushan S N over 4 yearsPlease reformat your code.
import
statement has got excluded from code