Write to, and read binary data from XML file in .Net 2.0
Solution 1
You seem to write some bad XML - use the following to write the data:
w.WriteStartDocument();
w.WriteStartElement("pstartdata");
#region Main Data
w.WriteElementString("pdata", "some data here");
// Write the binary data
w.WriteStartElement("bindata");
w.WriteBase64(fileData[1], 0, fileData[1].Length);
w.WriteEndElement();
#endregion (End) Main Data (End)
w.WriteEndDocument();
w.Flush();
fs.Close();
For reading you will have to use XmlReader.ReadContentAsBase64
.
As you have asked for other methods to write and read binary data - there are XmlTextWriter.WriteBinHex
and XmlReader.ReadContentAsBinHex
. BEWARE that these produce longer data than their Base64 pendants...
Solution 2
To read data from XML, you can use an XmlReader
, specifically the ReadContentAsBase64
method.
Another alternative is to use Convert.FromBase64String
:
byte[] binaryData;
try
{
binaryData = System.Convert.FromBase64String(base64String);
}
Note: you should look at the code your are using for writing - it appears that you are writing the binary data into the pstartdata
element (as well as a pdata
element beforehand). Doesn't look quite right - see the answer from @Yahia.
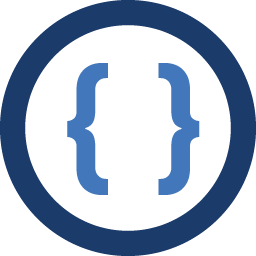
Admin
Updated on July 13, 2022Comments
-
Admin almost 2 years
I've ready many posts (such as this one) on SO now which explain how to write binary data to an XML using the XMLWriter.WriteBase64 method. However, I've yet to see one which explains how to then read the base64'd data. Is there another built in method? I'm also having a hard time finding solid documentation on the subject.
Here is the XML file that I'm using:
<?xml version="1.0"?> <pstartdata> <pdata>some data here</pdata> emRyWVZMdFlRR0FFQUNoYUwzK2dRUGlBS1ZDTXdIREF ..... and much, much more. </pstartdata>
The C# code which creates that file (.Net 4.0):
FileStream fs = new FileStream(Path.GetTempPath() + "file.xml", FileMode.Create); System.Xml.XmlTextWriter w = new System.Xml.XmlTextWriter(fs, null); w.Formatting = System.Xml.Formatting.None; w.WriteStartDocument(); w.WriteStartElement("pstartdata"); #region Main Data w.WriteElementString("pdata", "some data here"); // Write the binary data w.WriteBase64(fileData[1], 0, fileData[1].Length); #endregion (End) Main Data (End) w.WriteEndDocument(); w.Flush(); fs.Close();
Now, for the real challenge... Alright, so as you all can see the above was written in .Net 4.0. Unfortunately, the XML file needs to be read by an application using .Net 2.0. Reading in the binary (base 64) data has proven to be quite the challenge.
Code to read in XML data (.Net 2.0):
System.Xml.XmlDocument xDoc = new System.Xml.XmlDocument(); xDoc.LoadXml(xml_data); foreach (System.Xml.XmlNode node in xDoc.SelectNodes("pstartdata")) { foreach (System.Xml.XmlNode child in node.ChildNodes) { MessageBox.Show(child.InnerXml); } }
What would I need to add in order to read in the base 64'd data (shown above)?
-
Admin about 12 yearsThe difficult part for me is just loading the data in general as it's not directly part of an element string as the other data is. Understand what I'm getting at?
-
Oded about 12 years@Evan - See the answer from Yahia for that bit - you are writing all your data into the
pstartdata
element. Rethink how you are writing the data so it goes into one element only, to make reading it back out that much easier. -
O. R. Mapper over 10 yearsThe casual statement about using
XmlReader.ReadContentAsBase64
swiftly hides that using that method is not very straightforward unless you know how long the data is going to be. With that in mind, it seems that Oded's answer is a better description how to solve the problem. -
O. R. Mapper over 10 years+1, as the
Convert.FromBase64String
solution allows retrieving data of an unknown length in one call, as opposed to theReadContentAsBase64
method.