Write to JSON file using CodeIgniter
You are looping the countries object as an array. Have a look at this
<?php
class European_countries extends CI_Controller {
public function __construct()
{
parent::__construct();
$this->load->model('European_countries_model');
$this->load->helper('file');
$this->load->database();
}
public function index()
{
$countries = $this->European_countries_model->get_countries();
$data['countries'] = $countries;
$response = array();
$posts = array();
foreach ($countries as $country)
{
$posts[] = array(
"title" => $country->euro_id,
"flag" => $country->flag_name,
"population" => $country->population,
"avg_annual_gcountryth" => $country->avg_annual_gcountryth,
"date" => $country->date
);
}
$response['posts'] = $posts;
echo json_encode($response,TRUE);
//If the json is correct, you can then write the file and load the view
// $fp = fopen('./eur_countries_array.json', 'w');
// fwrite($fp, json_encode($response));
// if ( ! write_file('./eur_countries_array.json', $arr))
// {
// echo 'Unable to write the file';
// }
// else
// {
// echo 'file written';
// }
// $this->load->view('european_countries_view', $data);
}}?>
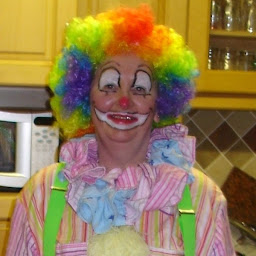
Pamela Keogh
Updated on June 04, 2022Comments
-
Pamela Keogh almost 2 years
I'm very new to php, mySql and CodeIgniter. I'm trying to read from mySql file and write the data read to a JSON file using CodeIgniter. I can read the data from mySql and view it and can create JSON file but have gotten totally bogged down with all the different information I'm reading on internet. I've been searching all night to see where my code for writing to JSON even goes, ie Controller, View, Model etc.
This is "European_countries_model.php"
// Model to access database class European_countries_model extends CI_Model { public function __construct() { $this->load->database(); } public function get_countries() { // get data from table "european_countries" $query = $this->db->get('european_countries'); return $query->result(); } }
This is my view which works (set up to see if it was reading database ok):
<?php // TEST - display data echo "Euopean Countries<br/>"; foreach ($countries as $country){ echo $country->euro_id." ".$country->title." ".$country->flag_name." ".$country->population." ".$country->avg_annual_growth." ".$country->date."<br/>"; } ?>
This is my controller "European_countries.php"
<?php // European countries controller class European_countries extends CI_Controller { public function __construct() { parent::__construct(); $this->load->model('European_countries_model'); // file helper contains functions that assist in working with files $this->load->helper('file'); $this->load->database(); } // pass all countries from model to view???? public function index() { $data['countries'] = $this->European_countries_model->get_countries(); //$this->load->view('european_countries_view', $data); $response = array(); $posts = array(); foreach ($countries as $country) { $title=$country['euro_id']; $flag=$country['flag_name']; $population=$country['population']; $avg_annual_gcountryth=$country['avg_annual_gcountryth']; $date=$country['date']; $posts[] = array('title'=> $title, 'flag_name'=> $flag_name, 'population'=>$population, 'avg_annual_gcountryth'=>$avg_annual_gcountryth, 'date'=>$date ); } $response['posts'] = $posts; $fp = fopen('./eur_countries_array.json', 'w'); fwrite($fp, json_encode($response)); /* if ( ! write_file('./eur_countries_array.json', $arr)) { echo 'Unable to write the file'; } else { echo 'file written'; } */ } } ?>
I think I've gone from following php to mySql to CodeIgniter and back again so many times, I've completely messed it up. Any help appreciated.
-
Pamela Keogh almost 9 yearsHi Carlos, Yeehaa! that's it :-). Thank you very much for taking the time to help me out. Much appreciated. Pamela