Write to XML in ruby
31,982
Solution 1
Builder should probably be your first stopping point:
require 'builder'
def product_xml
xml = Builder::XmlMarkup.new( :indent => 2 )
xml.instruct! :xml, :encoding => "ASCII"
xml.product do |p|
p.name "Test"
end
end
puts product_xml
produces this:
<?xml version="1.0" encoding="ASCII"?>
<product>
<name>Test</name>
</product>
which looks about right to me.
Some Builder references:
Solution 2
Simply with Nokogiri::XML::Builder
require 'nokogiri'
builder = Nokogiri::XML::Builder.new(:encoding => 'UTF-8') do |xml|
xml.root {
xml.products {
xml.widget {
xml.id_ "10"
xml.name "Awesome widget"
}
}
}
end
puts builder.to_xml
Will output:
<?xml version="1.0" encoding="UTF-8"?>
<root>
<products>
<widget>
<id>10</id>
<name>Awesome widget</name>
</widget>
</products>
</root>
Solution 3
You can use builder to generate xml.
Solution 4
Here are a couple more options for constructing XML in Ruby
REXML - built-in but is very slow especially when dealing with large documents
Nokogiri - newer and faster, installs as a rubygem
LibXML-Ruby - built on the C libxml library, also installs as a rubygem
If you can't install rubygems then REXML is your best options. If you are going to be creating large complex XML docs then Nokogiri or LibXML-Ruby is what you would want to use.
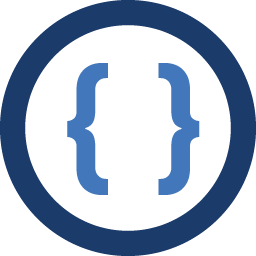
Author by
Admin
Updated on February 23, 2020Comments
-
Admin over 4 years
I am completely new to Ruby. All I want is to produce a simple XML file.
<?xml version="1.0" encoding="ASCII"?> <product> <name>Test</name> </product>
That's it.
-
ocodo about 13 yearsAll these are really for parsing existing xml, you should use Builder to create xml.
-
froderik over 12 yearsnokogiri got a builder also - not sure if it is any better but if you are doing both parsing and writing it may be sweet to use the same lib.
-
PatrickWalker over 11 yearsNokogiris builder seems focused on blocks. It does work but I've found Builder very helpful with building xml programatically
-
FilBot3 about 8 yearsThis is probably the most simple example of Nokogiri I've seen yet. Awesome.
-
pioto over 6 yearsThe API docs link seems to be dead (I think rubyforge.org itself is no more?). Is there an updated link available?
-
David Tresner-Kirsch over 6 years@pioto docs are available at github.com/jimweirich/builder -- I've proposed an edit fixing the link in the original post, but it's pending review.