Writing a Python list of lists to a csv file
Solution 1
Python's built-in CSV module can handle this easily:
import csv
with open("output.csv", "wb") as f:
writer = csv.writer(f)
writer.writerows(a)
This assumes your list is defined as a
, as it is in your question. You can tweak the exact format of the output CSV via the various optional parameters to csv.writer()
as documented in the library reference page linked above.
Update for Python 3
import csv
with open("out.csv", "w", newline="") as f:
writer = csv.writer(f)
writer.writerows(a)
Solution 2
You could use pandas
:
In [1]: import pandas as pd
In [2]: a = [[1.2,'abc',3],[1.2,'werew',4],[1.4,'qew',2]]
In [3]: my_df = pd.DataFrame(a)
In [4]: my_df.to_csv('my_csv.csv', index=False, header=False)
Solution 3
import csv
with open(file_path, 'a') as outcsv:
#configure writer to write standard csv file
writer = csv.writer(outcsv, delimiter=',', quotechar='|', quoting=csv.QUOTE_MINIMAL, lineterminator='\n')
writer.writerow(['number', 'text', 'number'])
for item in list:
#Write item to outcsv
writer.writerow([item[0], item[1], item[2]])
official docs: http://docs.python.org/2/library/csv.html
Solution 4
Using csv.writer in my very large list took quite a time. I decided to use pandas, it was faster and more easy to control and understand:
import pandas
yourlist = [[...],...,[...]]
pd = pandas.DataFrame(yourlist)
pd.to_csv("mylist.csv")
The good part you can change somethings to make a better csv file:
yourlist = [[...],...,[...]]
columns = ["abcd","bcde","cdef"] #a csv with 3 columns
index = [i[0] for i in yourlist] #first element of every list in yourlist
not_index_list = [i[1:] for i in yourlist]
pd = pandas.DataFrame(not_index_list, columns = columns, index = index)
#Now you have a csv with columns and index:
pd.to_csv("mylist.csv")
Solution 5
If for whatever reason you wanted to do it manually (without using a module like csv
,pandas
,numpy
etc.):
with open('myfile.csv','w') as f:
for sublist in mylist:
for item in sublist:
f.write(item + ',')
f.write('\n')
Of course, rolling your own version can be error-prone and inefficient ... that's usually why there's a module for that. But sometimes writing your own can help you understand how they work, and sometimes it's just easier.
Related videos on Youtube
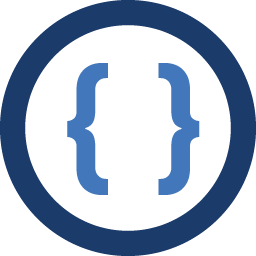
Admin
Updated on September 10, 2021Comments
-
Admin over 2 years
I have a long list of lists of the following form ---
a = [[1.2,'abc',3],[1.2,'werew',4],........,[1.4,'qew',2]]
i.e. the values in the list are of different types -- float,int, strings.How do I write it into a csv file so that my output csv file looks like
1.2,abc,3 1.2,werew,4 . . . 1.4,qew,2
-
Burhan Khalid over 11 yearsThis would get my +1 if you could explain your answer with some comments.
-
Amber over 11 years
writerow
does not take multiple arguments. -
Amber over 11 years>>> w.writerow("a", "b", "c") Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: writerow() takes exactly one argument (3 given)
-
Dmitry Zagorulkin over 11 years@Amber what version of python do you use?
-
Dmitry Zagorulkin over 11 years@Amber excuse me. i missed []
-
Vlad V almost 9 yearsFor Python 3 compatibility, remove the "b" from "wb".
-
Spas almost 9 yearsWith Python 3 - open('output.csv', 'w', newline=''). I get an extra line if I omit the newline parameter. docs.python.org/3/library/csv.html#csv.writer
-
Tim Mottram about 7 yearsIn python3 I had to use open('output.csv', 'w', newline="")
-
Cloud over 6 yearsI don't think should use
pandas
if built-in librarycsv
can do it. -
dorbodwolf over 6 yearsi like pandas because its powerful
-
Rambatino almost 6 yearsWOW that python 3 error is very unhelpful. Thanks @vladV (a bytes-like object is required, not 'str'). It kinda makes sense in hindsight, but not informative of where to look at all.
-
SDG almost 5 years@VladV I think your comment should be mentioned on Amber's answer.
-
Admin over 4 yearspandas is powerful, sure, but I'm not going to use a McLaren to drive to the corner store next door.
-
tlalco over 4 yearsIf you get error "TypeError: 'newline' is an invalid keyword argument for this function" just remove the newline argument. with open(file_name, "w") as f:
-
Amber over 4 years@tlalco that probably means you're using Python 2, in which case you should use the first code block rather than the second. (It also means you should consider switching to Python 3.)
-
anjandash over 2 yearsNote: For cases, where the list of lists is very large, sequential csv write operations can make the code significantly slower. In such cases using pandas would be wiser.