Xcode 4.2 iOS 5 : Multiple segues from a UITableView
Solution 1
Define two "generic" segues (identified as "segue1" and "segue2", for example) in the storyboard from your source view controller, one to each destination view controller. These segues won't be associated with any action.
Then, conditionally perform the segues in your UITableViewDelegate
:
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
// Conditionally perform segues, here is an example:
if (indexPath.row == 0)
{
[self performSegueWithIdentifier:@"segue1" sender:self];
}
else
{
[self performSegueWithIdentifier:@"segue2" sender:self];
}
}
Solution 2
I have the same problem as you do. The problem is that you can't link your tableViewCell to multiple view controllers. However you can link your source view itself to multiple view controllers.
Control-drag the master view controller (instead of table view cell) from the scene viewer to whatever view controller you want to link. You can do this as much as you want. Notice that the segue shown in source view controller scene should be something like "Push Segue from Root View Controller ..." instead of "Push Segue from NavCell to ...".
Identify each segue link a unique name like "toDetailView1"
Finally, custom the selection in your source view controllers:
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath { if (indexPath.row % 2 == 1) { [self performSegueWithIdentifier:@"toDetailView1" sender:self]; } else { [self performSegueWithIdentifier:@"toDetailView2" sender:self]; } }
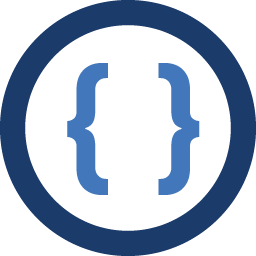
Admin
Updated on June 08, 2022Comments
-
Admin almost 2 years
I'm starting now with Xcode on 4.2 for iOS5 and there are a few changes and I'm now crossing a problem that I can't figure out a way to solve it.
I'm doing an example with a UITablwView that is populated programmatically with 2 Sections, 1st section with only 1 Row, and 2nd Section with 3 Rows.
My aim is to select a row from the table, and based on that row, the user will be redirected to different Views.
For example: selecting section 0 row 0, app pushes to view 1 - name setting // selecting section 1 row 0, app pushes to view 3 - address setting
The old fashion way, this is quite simple, just needed to init a UIViewController with initWithNibName and then push the view.
Now with the storyBoard everything changes, or at least I think it changes because I can't see how to get the same result since I can't set multiple segue's from the tableView to different UIViewControllers...and to do the old fashion way I can't see where I can get the NIB names from the views on the storyBoard to init an UIViewController to push.
Does any one knows how to get to this result??
-
mm24 about 10 yearsFantastic reply. Works for me as well. Should be accepted as answer.
-
Steve Sahayadarlin almost 10 yearsAmazing! Solved my problem! One question... How can I use the same thing for more than two segues. Let's say I have 7 tableView cells. How would I use that in addition to the code you provided?