XML: How to get Elements by Attribute Value - Python 2.7 and minidom
Solution 1
If you don't find a built-in method, why not iterate over the items?
from xml.dom import minidom
xmldoc = minidom.parse(r"C:\File.xml")
PFD = xmldoc.getElementsByTagName("PFD")
PNT = xmldoc.getElementsByTagName("PNT")
for element in PNT:
if element.getAttribute('A') == "1":
print "element found"
Adding the items to a list should be easy now.
Solution 2
If you aren't limited to using xml.dom.minidom, lxml has better search functionality. Note that lxml is not builtin, and will require installing the lxml package and non-Python dependencies.
Eg:
>>> from lxml import etree
>>> root = etree.parse(r"C:\File.xml")
>>> for e in root.findall('PNT[@A="1"]'):
... print etree.tostring(e)
<PNT A="1" B="c"/>
<PNT A="1" B="b"/>
Lxml also supports all of XPath via element.xpath('query')
. Other convenience functions include element.findtext
which finds the appropriate element and returns the text of it, element.find
and element.findall
which returns the first/list of all elements matching a query using a subset of XPath covering common queries.
Solution 3
Try this:
from xml.dom import minidom
xmldoc = minidom.parse(r"C:\File.xml")
PNT = xmldoc.getElementsByTagName("PNT")
for element in PNT:
print element.attributes.keys()
for elem in element.attributes.values():
print elem.firstChild.data
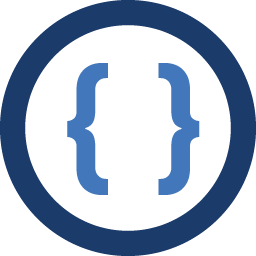
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I want to get a list of XML Elements based first on TagName and second on Attribute Value. I´m using the xml.dom library and python 2.7.
While it´s easy to get the first step done:
from xml.dom import minidom xmldoc = minidom.parse(r"C:\File.xml") PFD = xmldoc.getElementsByTagName("PFD") PNT = PFD.getElementsByTagName("PNT")
I´ve been looking around but cannot find a solution for the second step. Is there something like a
.getElementsByAttributeValue
that would give me a list to work with?If the XML looks like this
<PFD> <PNT A="1" B=.../> <PNT A="1" B=.../> <PNT A="2" B=.../> </PFD>
In need all PNTs where A="1" in a list.