XOR on two lists in Python
Solution 1
Given sequences seq1
and seq2
, you can calculate the symmetric difference with
set(seq1).symmetric_difference(seq2)
For example,
In [19]: set([1,2,5]).symmetric_difference([1,2,9,4,8,9])
Out[19]: {4, 5, 8, 9}
Tip: Generating the set with the smaller list is generally faster:
In [29]: %timeit set(range(60)).symmetric_difference(range(600))
10000 loops, best of 3: 25.7 µs per loop
In [30]: %timeit set(range(600)).symmetric_difference(range(60))
10000 loops, best of 3: 41.5 µs per loop
The reason why you may want to use symmetric difference
instead of ^
(despite the beauty of its syntax) is because the symmetric difference
method can take a list as input. ^
requires both inputs be sets. Converting both lists into sets is a little more computation than is minimally required.
This question has been marked of as a duplicate of this question That question, however, is seeking a solution to this problem without using sets.
The accepted solution,
[a for a in list1+list2 if (a not in list1) or (a not in list2)]
is not the recommended way to XOR two lists if sets are allowed. For one thing, it's over 100 times slower:
In [93]: list1, list2 = range(600), range(60)
In [94]: %timeit [a for a in list1+list2 if (a not in list1) or (a not in list2)]
100 loops, best of 3: 3.35 ms per loop
Solution 2
There's the XOR
operator on set. Assuming you have no duplicates (and you don't care about checking if an element appears more than 1 times or not in the second list), you can use the ^
operator on set:
>>> set([1, 2, 3, 4, 5]) ^ set([1, 3, 4, 5, 6])
set([2, 6])
>>> set(range(80)) ^ set(range(60))
set([60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79])
Related videos on Youtube
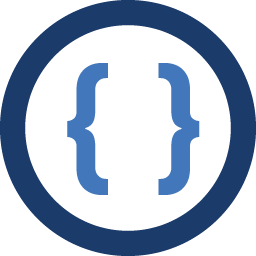
Admin
Updated on March 30, 2020Comments
-
Admin about 4 years
I'm a beginner in Python, and I have to do the XOR between two lists (the first one with the length : 600 and the other 60)
I really don't know how to do that, if somebody can explain me how, it will be a pleasure.
I have to do that to find the BPSK signal module, and I'm wondering on how doing that with two lists that haven't the same length. I saw this post : Comparing two lists and only printing the differences? (XORing two lists) but the length of lists is the same
Thanks for your help, and sry for my bad english Rom
-
Kevin Johnson about 10 years
-
unutbu about 10 yearsNote that question stackoverflow.com/questions/16312730/… has the extra requirement that sets can not be used. This question does not impose that requirement. Simpler and faster solutions are possible if sets are allowed.
-
-
holzkohlengrill over 7 yearsgreat and thorough answer!