Xpath error has an Invalid Token
Solution 1
Notice the apostrophe ('
) in codedesc
?
You need to escape it somehow. The XPath interpreter considers it as a string delimiter and doesn't know how to treat the other apostrophe after it.
One way you can do that is by enclosing your string in double quotes instead of apostrophes.
Your code could therefore become:
var selectNode = xmlDoc.SelectSingleNode(
"//CodeType[@name='" + codetype + "']" +
"/Section[@title='" + section + "']" +
"/Code[@code=\"" + code + "' and @description='" + codedesc + "\"]")
as XmlElement;
(note that on the fourth line, the apostrophes('
) became double quotes(\"
))
While this approach works for the data you presented, you are still not 100% safe: other records could contain double quotes themselves. If that happens, we'll need to think of something for that case as well.
Solution 2
You can get selected node based on index , if any special characters in the xml schema. So , here looks below implementation for delete selected index node from xml schema.
XML SelectSingleNode Delete Operation
var schemaDocument = new XmlDocument();
schemaDocument.LoadXml(codesXML);
var xmlNameSpaceManager = new XmlNamespaceManager(schemaDocument.NameTable);
if (schemaDocument.DocumentElement != null)
xmlNameSpaceManager.AddNamespace("x", schemaDocument.DocumentElement.NamespaceURI);
var codesNode = schemaDocument.SelectSingleNode(@"/x:integration-engine-codes/x:code-categories/x:code-category/x:codes", xmlNameSpaceManager);
var codeNode = codesNode.ChildNodes.Item(Convert.ToInt32(index) - 1);
if (codeNode == null || codeNode.ParentNode == null)
{
throw new Exception("Invalid node found");
}
codesNode.RemoveChild(codeNode);
return schemaDocument.OuterXml;
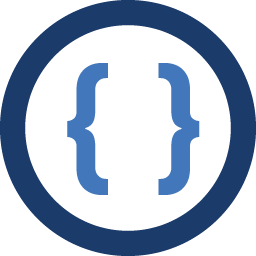
Admin
Updated on June 13, 2020Comments
-
Admin almost 4 years
I have the following C# code:
var selectNode = xmlDoc.SelectSingleNode("//CodeType[@name='" + codetype + "']/Section[@title='" + section + "']/Code[@code='" + code + "' and @description='" + codedesc + "']") as XmlElement;
when I run my code it raises the error saying "the above statement has an invalid token"
These are the values for the above statement.
codeType=cbc section="Mental" codedesc="Injection, enzyme (eg, collagenase), palmar fascial cord (ie, Dupuytren's contracture"
-
Admin almost 12 yearsThanks so much, I will find way to get ride of the apostrophe in my codedesc data.