Yii upload a file using a model
16,144
CFormModel
doesn't have a method named save()
, if you want to call it you have to implement it, but what you want in your case is to use the validate
method
And if MyFile
doesn't have a related db table then it shouldn't extend CActiveRecord
.
You can validate that the uploaded image is a gif, a png or a jpg by calling validate()
:
class MyFileController extends CController {
public function actionCreate() {
$model = new MyFile;
if(isset($_POST['MyFile'])) {
$model->attributes=$_POST['MyFile'];
$model->image=CUploadedFile::getInstance($model,'image');
if($model->validate()) {
//The image is valid, you can save it
$path = Yii::app()->runtimePath.'/temp/uploadDirectory/'.$model->image;
$model->image->saveAs($path);
}
$this->render('create', array('model'=>$model));
}
}
}
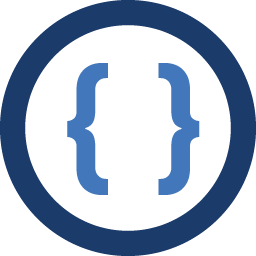
Author by
Admin
Updated on August 21, 2022Comments
-
Admin over 1 year
I am simply trying to upload a file using a model. I get the exception message in the current situation (see model/controller/view below):
CException MyFile and its behaviors do not have a method or closure named "save".
If my model extends CActiveRecord instead of CFormModel there is an another Exception:
CDbException The table "MyFile" for active record class "MyFile" cannot be found in the database.
What is my mistake? These are the files:
MODEL: MyFile.php
class MyFile extends CFormModel { public $image; public function rules () { return array ( array ('image', 'file', 'types' => 'gif, jpg, png'), ); } }
CONTROLLER: MyFileController.php
class MyFileController extends CController { public function actionCreate() { $model = new MyFile; if(isset($_POST['MyFile'])) { $model->attributes=$_POST['MyFile']; $model->image=CUploadedFile::getInstance($model,'image'); if($model->save()) { $path = Yii::app()->runtimePath.'/temp/uploadDirectory/'.$model->image; $model->image->saveAs($path); } } $this->render('create', array('model'=>$model)); } }
VIEW: create.php
<h1>File-Upload</h1> <?php echo CHtml::form('','post',array('enctype'=>'multipart/form-data')); echo CHtml::activeFileField($model, 'image'); echo CHtml::submitButton('abschicken', array('name' => 'submit')); echo CHtml::endForm(); ?>
-
Admin over 10 yearsNow I have a table and I am using CActiveRecord. Now I have an another Exception: Missing the temporary folder to store the uploaded file "xyz.jpg".
-
darkheir over 10 yearsHum the folder
Yii::app()->runtimePath.'/temp/uploadDirectory/'
exists? if not then you should create it before saving the image with something likemkdir($yourPath, 0775, true)
(true
is to recursively create the folders) -
Admin over 10 yearsyes, the directory exists, the same error message.. I think, I must set the temporary directory somewhere in the setting, but I currently do not know, where?
-
Admin over 10 yearsI've found it: On the test-server there was no entry in the php.ini file at "upload_tmp_dir". I've changed it to /var/tmp
-
Jair almost 8 yearsuse this to store the file uploaded using Yii 1