Youtube iFrame embed stopVideo not a function
Solution 1
// https://developers.google.com/youtube/iframe_api_reference
// global variable for the player
var player;
// this function gets called when API is ready to use
function onYouTubePlayerAPIReady() {
// create the global player from the specific iframe (#video)
player = new YT.Player('video', {
events: {
// call this function when player is ready to use
'onReady': onPlayerReady
}
});
}
function onPlayerReady(event) {
// bind events
var playButton = document.getElementById("play-button");
playButton.addEventListener("click", function() {
player.playVideo();
});
var pauseButton = document.getElementById("pause-button");
pauseButton.addEventListener("click", function() {
player.pauseVideo();
});
}
// Inject YouTube API script
var tag = document.createElement('script');
tag.src = "//www.youtube.com/player_api";
var firstScriptTag = document.getElementsByTagName('script')[0];
firstScriptTag.parentNode.insertBefore(tag, firstScriptTag);
please check this example http://codepen.io/marti1125/pen/XdyPOe
Solution 2
Right, so you'll indeed need to load the iframe embed using the javascript API provided by YouTube. That might not work well with your lightbox however, as it seems to want an html string for its content.
Alternatively, you could always remove your youtube iframe on close - that definitely will stop the video/audio. I suspect setting your lightbox content to an empty string ("") or even an empty div or iframe should do the trick...
Hope this helps!
Related videos on Youtube
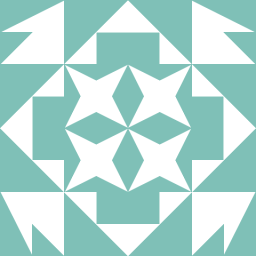
user3861559
Updated on June 04, 2022Comments
-
user3861559 12 months
I am trying to embed a youtube iframe in a custom light box I have on my site. The embed itself works fine. The lightbox closes when I attempt to close it but the video (audio rather) keeps play in the background.
stopVideo
function returns "stopVideo is not a function"$("#youTubeLink").click(function(){ var f = '<iframe id="ytplayer" type="text/html" width="100%" height="400px" src="http://www.youtube.com/embed/M7lc1UVf-VE?enablejsapi=1&origin=http://example.com" frameborder="0"></iframe>' global.addLightboxContent(f); global.showLightbox(); });
When I close the lightbox this is what happens
$("div#lightbox-close").click(function() { $('#ytplayer').stopVideo(); global.killLightbox(); }
- global lightbox functions are doing what they should (add iframe into LB, show it and then kill it)
- when
div#lightbox-close
is clicked on, the function is triggered. Tested with a console log
I am guessing I am missing some sort of Youtube js script include. I am not sure what exactly.
-
user3861559 over 6 yearsHey! Really appreciate the first paragraph. That was really helpful in understanding the root cause. I am now doing .remove() on the iframe on lightbox close. That's working for now. Thanks.
-
nibnut over 6 yearsSure thing. Any time.