Zend framework $db->update result
11,222
Solution 1
$data = array(
'updated_on' => '2007-03-23',
'bug_status' => 'FIXED',
);
$n = 0;
try {
$n = $db->update('bugs', $data, 'bug_id = 2');
} catch (Zend_Exception $e) {
die('Something went wrong: ' . $e->getMessage());
}
if (empty($n)) {
die('Zero rows affected');
}
Solution 2
If you are just looking for a boolean return value, this solution is the best for handling success in the model:
class Default_Model_Test extends Zend_Db_Table {
public function updateTest($testId, $testData){
try {
$this->_db->update('test', $testData, array(
'id = ?' => $testId
));
return true;
}
catch (Exception $exception){
return false;
}
}
}
But, a better solution would be to handling success from the controller level, because it is making the request:
class Default_Model_Test extends Zend_Db_Table {
public function updateTest($testId, $testData){
$this->_db->update('test', $testData, array(
'id = ?' => $testId
));
}
}
class Default_TestController extends Zend_Controller_Action {
public function updateAction(){
try {
$testId = $this->_request->getParam('testId');
if (empty($testId)) throw new Zend_Argument_Exception('testId is empty');
$testData = $this->_request->getPost();
if (empty($testId)) throw new Zend_Argument_Exception('testData is empty');
$testModel->updateTest($testId, $testData);
}
catch (Exception $exception){
switch (get_class($exception)){
case 'Zend_Argument_Exception': $message = 'Argument error.'; break;
case 'Zend_Db_Statement_Exception': $message = 'Database error.'; break;
case default: $message = 'Unknown error.'; break;
}
}
}
}
This is an excellent solution when working with multiple resource types, using a switch on the exception type, and doing what is appropriate based on your program's needs. Nothing can escape this vacuum.
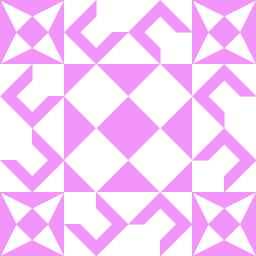
Author by
Handsome Nerd
Updated on June 04, 2022Comments
-
Handsome Nerd 7 months
Zend_Db_Adapter::update()
returns the number of rows affected by the update operation. What is best way to determine if the query was successful?$data = array( 'updated_on' => '2007-03-23', 'bug_status' => 'FIXED' ); $n = $db->update('bugs', $data, 'bug_id = 2');